Part 2: Verification of Derivative Function#
To run this script you need output from Selecting and Reindexing of Area of Interest.
In Part 1: Deriving Derivatives on Triangular Grid we introduced a derivative()
function to calculate derivatives on a triangular grid. Since it is difficult to tell with the naked eye whether our function did what we wanted it to do, we create an artificially generated field that has a constant gradient along one axis and remains constant along the other axis.
import numpy as np
import xarray as xr
Range of area of interest:
# Max Plancks birthplace, Kiel, Schleswig-Holstein, Germany
left_bound = 9.88
right_bound = 10.38
top_bound = 54.57
bottom_bound = 54.07
We import the selected_indices
, hence the indices for cells
, vertices
and edges
, just like the new_grid
which contains the grid information of the area of interest.
selected_indices = xr.open_dataset(
f"../selected_indices_region_{bottom_bound}-{top_bound}_{left_bound}-{right_bound}.nc"
)
new_grid = xr.open_dataset(
f"../new_grid_region_{bottom_bound}-{top_bound}_{left_bound}-{right_bound}.nc"
)
new_grid
<xarray.Dataset> Dimensions: (cell: 70, nv: 3, vertex: 50, ne: 6, edge: 119, no: 4, nc: 2, max_stored_decompositions: 4, two_grf: 2, cell_grf: 14, max_chdom: 1, edge_grf: 24, vert_grf: 13) Coordinates: clon (cell) float64 0.1804 0.1805 ... 0.174 clat (cell) float64 0.9453 0.946 ... 0.9488 vlon (vertex) float64 0.1815 0.1807 ... 0.1717 vlat (vertex) float64 0.9456 0.9466 ... 0.9482 elon (edge) float64 0.1811 0.1814 ... 0.1724 elat (edge) float64 0.9461 0.9471 ... 0.9487 * cell (cell) int64 4282376 4282377 ... 4283130 * vertex (vertex) int32 2144929 2144932 ... 2145286 * edge (edge) int32 6427302 6427311 ... 6428420 Dimensions without coordinates: nv, ne, no, nc, max_stored_decompositions, two_grf, cell_grf, max_chdom, edge_grf, vert_grf Data variables: (12/91) clon_vertices (cell, nv) float64 0.1794 0.1802 ... 0.173 clat_vertices (cell, nv) float64 0.9457 0.9446 ... 0.9492 vlon_vertices (vertex, ne) float64 9.969e+36 ... 9.969e+36 vlat_vertices (vertex, ne) float64 9.969e+36 ... 9.969e+36 elon_vertices (edge, no) float64 0.1807 0.1805 ... 0.1728 elat_vertices (edge, no) float64 0.9466 0.946 ... 0.9485 ... ... edge_dual_normal_cartesian_x (edge) float64 -0.6706 -0.7373 ... -0.7362 edge_dual_normal_cartesian_y (edge) float64 -0.5071 0.4974 ... 0.4973 edge_dual_normal_cartesian_z (edge) float64 0.5414 0.4572 ... 0.4589 cell_circumcenter_cartesian_x (cell) float64 0.576 0.5755 ... 0.5739 cell_circumcenter_cartesian_y (cell) float64 0.105 0.105 ... 0.1003 0.1009 cell_circumcenter_cartesian_z (cell) float64 0.8107 0.8111 ... 0.8127 Attributes: (12/43) title: ICON grid description institution: Max Planck Institute for Meteorology/Deutscher ... source: git@git.mpimet.mpg.de:GridGenerator.git revision: d00fcac1f61fa16c686bfe51d1d8eddd09296cb5 date: 20180529 at 222250 user_name: Rene Redler (m300083) ... ... topography: modified SRTM30 subcentre: 1 number_of_grid_used: 15 history: Thu Aug 16 11:05:44 2018: ncatted -O -a ICON_gr... ICON_grid_file_uri: http://icon-downloads.mpimet.mpg.de/grids/publi... NCO: netCDF Operators version 4.7.5 (Homepage = http...
- cell: 70
- nv: 3
- vertex: 50
- ne: 6
- edge: 119
- no: 4
- nc: 2
- max_stored_decompositions: 4
- two_grf: 2
- cell_grf: 14
- max_chdom: 1
- edge_grf: 24
- vert_grf: 13
- clon(cell)float64...
- long_name :
- center longitude
- units :
- radian
- standard_name :
- grid_longitude
- bounds :
- clon_vertices
array([0.180381, 0.180509, 0.179222, 0.178862, 0.178989, 0.177701, 0.17975 , 0.181041, 0.180912, 0.180152, 0.176936, 0.178098, 0.176043, 0.17681 , 0.178463, 0.177575, 0.178336, 0.179622, 0.179979, 0.181136, 0.179096, 0.179518, 0.178625, 0.17939 , 0.180686, 0.179921, 0.180815, 0.18005 , 0.178752, 0.177332, 0.177458, 0.176168, 0.178225, 0.180281, 0.173859, 0.172969, 0.1759 , 0.177054, 0.175012, 0.174369, 0.175526, 0.173477, 0.174246, 0.176291, 0.175136, 0.177179, 0.176416, 0.174758, 0.17565 , 0.174882, 0.1736 , 0.173091, 0.17794 , 0.173214, 0.174111, 0.173337, 0.17256 , 0.175791, 0.174625, 0.176689, 0.173725, 0.175398, 0.174501, 0.175273, 0.176563, 0.177984, 0.177857, 0.172828, 0.172705, 0.173987])
- clat(cell)float64...
- long_name :
- center latitude
- units :
- radian
- standard_name :
- grid_latitude
- bounds :
- clat_vertices
array([0.945285, 0.945958, 0.945008, 0.947452, 0.948124, 0.947173, 0.947041, 0.947991, 0.947319, 0.948402, 0.948256, 0.948535, 0.948666, 0.947584, 0.946091, 0.946501, 0.945418, 0.946369, 0.943924, 0.944201, 0.944334, 0.950157, 0.950568, 0.949486, 0.950435, 0.951518, 0.951106, 0.952188, 0.951239, 0.949618, 0.950289, 0.949338, 0.949207, 0.949074, 0.944315, 0.944723, 0.944186, 0.944466, 0.944595, 0.946351, 0.946631, 0.94676 , 0.945678, 0.945548, 0.945269, 0.945139, 0.946221, 0.947713, 0.947304, 0.948385, 0.947432, 0.945397, 0.944056, 0.949877, 0.949467, 0.950548, 0.951629, 0.951782, 0.951501, 0.951371, 0.951911, 0.950419, 0.95083 , 0.949748, 0.9507 , 0.952321, 0.951651, 0.948514, 0.947841, 0.948795])
- vlon(vertex)float64...
- long_name :
- vertex longitude
- units :
- radian
- standard_name :
- grid_longitude
- bounds :
- vlon_vertices
array([0.181467, 0.18071 , 0.182221, 0.182002, 0.179422, 0.18018 , 0.178138, 0.179951, 0.177899, 0.178662, 0.179189, 0.176613, 0.181244, 0.177134, 0.175846, 0.175077, 0.177377, 0.180935, 0.178897, 0.181781, 0.181018, 0.180253, 0.179719, 0.178425, 0.180483, 0.177657, 0.178953, 0.176365, 0.172778, 0.174818, 0.174052, 0.172008, 0.176858, 0.176095, 0.175331, 0.174563, 0.173284, 0.172513, 0.173793, 0.172245, 0.171467, 0.173531, 0.17302 , 0.174305, 0.172754, 0.178184, 0.174821, 0.175594, 0.176887, 0.171739])
- vlat(vertex)float64...
- long_name :
- vertex latitude
- units :
- radian
- standard_name :
- grid_latitude
- bounds :
- vlat_vertices
array([0.945555, 0.946639, 0.944471, 0.947588, 0.945688, 0.944605, 0.944737, 0.947722, 0.947854, 0.946771, 0.948805, 0.946902, 0.948671, 0.948937, 0.947985, 0.949067, 0.94582 , 0.943521, 0.943653, 0.950703, 0.951786, 0.952869, 0.950837, 0.949887, 0.949754, 0.95097 , 0.95192 , 0.950019, 0.944041, 0.943914, 0.944996, 0.945123, 0.943784, 0.944867, 0.94595 , 0.947032, 0.946078, 0.94716 , 0.948114, 0.950276, 0.951357, 0.951229, 0.949195, 0.950148, 0.95231 , 0.953002, 0.952182, 0.9511 , 0.952052, 0.948241])
- elon(edge)float64...
- long_name :
- edge midpoint longitude
- units :
- radian
- standard_name :
- grid_longitude
- bounds :
- elon_vertices
array([0.181089, 0.181356, 0.180445, 0.179801, 0.180823, 0.180066, 0.17878 , 0.179159, 0.181201, 0.178925, 0.178281, 0.179306, 0.17957 , 0.178544, 0.177256, 0.177638, 0.180331, 0.179686, 0.180977, 0.181623, 0.180597, 0.180217, 0.177517, 0.17649 , 0.176873, 0.178161, 0.176106, 0.175462, 0.17623 , 0.178019, 0.1784 , 0.179042, 0.176995, 0.177758, 0.181578, 0.180558, 0.179538, 0.179916, 0.178518, 0.180636, 0.1814 , 0.179072, 0.179454, 0.180102, 0.178688, 0.178041, 0.178807, 0.179836, 0.18075 , 0.181132, 0.180368, 0.179986, 0.179337, 0.179603, 0.178305, 0.177395, 0.17675 , 0.177779, 0.177011, 0.175721, 0.180864, 0.173798, 0.173415, 0.174435, 0.172393, 0.17303 , 0.175838, 0.176477, 0.175456, 0.177497, 0.177117, 0.175074, 0.174947, 0.173923, 0.174307, 0.175971, 0.175588, 0.173538, 0.172899, 0.174691, 0.173668, 0.175713, 0.176736, 0.176354, 0.175204, 0.17482 , 0.174179, 0.174435, 0.173153, 0.172646, 0.177877, 0.172888, 0.172499, 0.172633, 0.173663, 0.173275, 0.174049, 0.174692, 0.173919, 0.17211 , 0.173143, 0.175208, 0.17624 , 0.175854, 0.174176, 0.174563, 0.176626, 0.177273, 0.173787, 0.17495 , 0.175336, 0.17598 , 0.17792 , 0.178569, 0.177535, 0.172126, 0.172766, 0.173407, 0.172379])
- elat(edge)float64...
- long_name :
- edge midpoint latitude
- units :
- radian
- standard_name :
- grid_latitude
- bounds :
- elat_vertices
array([0.946097, 0.947113, 0.945622, 0.945146, 0.94508 , 0.946163, 0.945213, 0.944671, 0.944538, 0.947788, 0.947313, 0.947247, 0.948263, 0.94833 , 0.947378, 0.946837, 0.94718 , 0.946705, 0.947655, 0.94813 , 0.948197, 0.948738, 0.948395, 0.948461, 0.94792 , 0.948871, 0.949002, 0.948526, 0.947444, 0.946296, 0.945754, 0.94623 , 0.946361, 0.945278, 0.943996, 0.944063, 0.944129, 0.943587, 0.944195, 0.952327, 0.951245, 0.950362, 0.949821, 0.950296, 0.950904, 0.950429, 0.949346, 0.94928 , 0.95077 , 0.950229, 0.951312, 0.951853, 0.951378, 0.952394, 0.951445, 0.949953, 0.949478, 0.949412, 0.950494, 0.949543, 0.949213, 0.943978, 0.944519, 0.944455, 0.944582, 0.94506 , 0.943849, 0.944326, 0.944391, 0.944261, 0.944802, 0.944932, 0.946491, 0.946555, 0.946014, 0.946426, 0.946967, 0.947096, 0.946619, 0.945473, 0.945537, 0.945409, 0.945344, 0.945885, 0.947508, 0.948049, 0.947573, 0.94859 , 0.947637, 0.945601, 0.943719, 0.950753, 0.951293, 0.949736, 0.949672, 0.950212, 0.949131, 0.949607, 0.950689, 0.951834, 0.95177 , 0.951641, 0.951576, 0.952117, 0.951706, 0.951165, 0.951035, 0.951511, 0.952246, 0.950624, 0.950084, 0.95056 , 0.951986, 0.952461, 0.952527, 0.9477 , 0.948178, 0.948654, 0.948718])
- cell(cell)int644282376 4282377 ... 4283129 4283130
array([4282376, 4282377, 4282378, 4282400, 4282401, 4282402, 4282403, 4282404, 4282405, 4282407, 4282408, 4282409, 4282410, 4282411, 4282412, 4282413, 4282414, 4282415, 4282420, 4282421, 4282422, 4282480, 4282481, 4282482, 4282483, 4282484, 4282485, 4282486, 4282487, 4282488, 4282489, 4282490, 4282491, 4282493, 4282497, 4282501, 4282508, 4282510, 4282511, 4282512, 4282513, 4282514, 4282515, 4282516, 4282517, 4282518, 4282519, 4282520, 4282521, 4282522, 4282523, 4282527, 4282558, 4282936, 4282938, 4282939, 4282941, 4282960, 4282961, 4282962, 4282967, 4282968, 4282969, 4282970, 4282971, 4282972, 4282973, 4283128, 4283129, 4283130])
- vertex(vertex)int322144929 2144932 ... 2145239 2145286
array([2144929, 2144932, 2144933, 2144935, 2144937, 2144938, 2144939, 2144953, 2144954, 2144955, 2144956, 2144957, 2144958, 2144959, 2144960, 2144961, 2144962, 2144963, 2144968, 2144978, 2144983, 2144984, 2145002, 2145003, 2145004, 2145005, 2145006, 2145007, 2145008, 2145010, 2145011, 2145014, 2145020, 2145021, 2145022, 2145023, 2145024, 2145025, 2145026, 2145217, 2145218, 2145220, 2145222, 2145223, 2145224, 2145231, 2145237, 2145238, 2145239, 2145286], dtype=int32)
- edge(edge)int326427302 6427311 ... 6428419 6428420
array([6427302, 6427311, 6427312, 6427313, 6427314, 6427315, 6427316, 6427317, 6427318, 6427352, 6427353, 6427354, 6427355, 6427356, 6427357, 6427358, 6427359, 6427360, 6427361, 6427362, 6427363, 6427365, 6427366, 6427367, 6427368, 6427369, 6427370, 6427371, 6427372, 6427373, 6427374, 6427375, 6427376, 6427377, 6427385, 6427387, 6427388, 6427389, 6427390, 6427425, 6427426, 6427482, 6427483, 6427484, 6427485, 6427486, 6427487, 6427488, 6427489, 6427490, 6427491, 6427492, 6427493, 6427494, 6427495, 6427496, 6427497, 6427498, 6427499, 6427500, 6427501, 6427504, 6427507, 6427508, 6427513, 6427516, 6427527, 6427528, 6427529, 6427531, 6427532, 6427533, 6427534, 6427535, 6427536, 6427537, 6427538, 6427539, 6427540, 6427541, 6427542, 6427543, 6427544, 6427545, 6427546, 6427547, 6427548, 6427549, 6427550, 6427553, 6427602, 6428151, 6428152, 6428160, 6428161, 6428162, 6428164, 6428165, 6428166, 6428167, 6428170, 6428202, 6428203, 6428204, 6428205, 6428206, 6428207, 6428208, 6428213, 6428214, 6428215, 6428216, 6428217, 6428218, 6428219, 6428410, 6428418, 6428419, 6428420], dtype=int32)
- clon_vertices(cell, nv)float64...
array([[0.179422, 0.18018 , 0.181467], [0.181467, 0.18071 , 0.179422], [0.179422, 0.178138, 0.18018 ], ..., [0.173793, 0.17302 , 0.171739], [0.171739, 0.172513, 0.173793], [0.173793, 0.175077, 0.17302 ]])
- clat_vertices(cell, nv)float64...
array([[0.945688, 0.944605, 0.945555], [0.945555, 0.946639, 0.945688], [0.945688, 0.944737, 0.944605], ..., [0.948114, 0.949195, 0.948241], [0.948241, 0.94716 , 0.948114], [0.948114, 0.949067, 0.949195]])
- vlon_vertices(vertex, ne)float64...
array([[9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36], [9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36], [9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36], ..., [9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36], [9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36], [9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36]])
- vlat_vertices(vertex, ne)float64...
array([[9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36], [9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36], [9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36], ..., [9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36], [9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36], [9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36]])
- elon_vertices(edge, no)float64...
array([[0.18071 , 0.180509, 0.181467, 0.18167 ], [0.182002, 0.180912, 0.18071 , 0.181799], [0.181467, 0.180509, 0.179422, 0.180381], ..., [0.171739, 0.172705, 0.173793, 0.172828], [0.173793, 0.173987, 0.17302 , 0.172828], [0.17302 , 0.171931, 0.171739, 0.172828]])
- elat_vertices(edge, no)float64...
array([[0.946639, 0.945958, 0.945555, 0.946235], [0.947588, 0.947319, 0.946639, 0.946908], [0.945555, 0.945958, 0.945688, 0.945285], ..., [0.948241, 0.947841, 0.948114, 0.948514], [0.948114, 0.948795, 0.949195, 0.948514], [0.949195, 0.948923, 0.948241, 0.948514]])
- ifs2icon_cell_grid(cell)float64...
- long_name :
- ifs to icon cells
array([9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36])
- ifs2icon_edge_grid(edge)float64...
- long_name :
- ifs to icon edge
array([9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36])
- ifs2icon_vertex_grid(vertex)float64...
- long_name :
- ifs to icon vertex
array([9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36, 9.96921e+36])
- cell_area(cell)float64...
- long_name :
- area of grid cell
- units :
- m2
- standard_name :
- area
- grid_type :
- unstructured
- number_of_grid_in_reference :
- 1
array([25122696.100735, 25115307.267907, 25116300.534128, 25123237.931024, 25115830.247435, 25116842.75659 , 25115578.910138, 25114562.040095, 25121960.932241, 25122212.059399, 25117083.448929, 25123478.41463 , 25124729.658542, 25124499.793522, 25116581.784901, 25124249.665799, 25123979.275508, 25122977.159648, 25115999.004667, 25122394.754638, 25123688.622955, 25115025.063522, 25122653.375085, 25122442.873669, 25121393.215094, 25121593.997027, 25113974.845723, 25114166.124292, 25115226.0792 , 25123698.610424, 25116272.006796, 25117303.861822, 25116061.279655, 25114803.717182, 25119795.715901, 25127478.523255, 25118545.371572, 25124967.236121, 25126230.550547, 25119085.469371, 25125506.966573, 25126749.018405, 25126499.890636, 25125247.219992, 25118825.534301, 25117570.619972, 25117841.200154, 25125746.475592, 25118091.526784, 25118321.599695, 25119325.176469, 25120065.233457, 25117279.786595, 25126164.780769, 25118531.418818, 25118720.984305, 25118890.296435, 25124078.139111, 25117683.849702, 25116462.429012, 25125297.678096, 25117503.995341, 25125128.600582, 25124939.260832, 25123898.518499, 25115406.764477, 25122843.563845, 25119544.655465, 25126977.933665, 25125965.746968])
- dual_area(vertex)float64...
- long_name :
- areas of dual hexagonal/pentagonal cells
- units :
- m2
- standard_name :
- area
array([50236704.122656, 50237267.527659, 50236100.118676, 50235230.038 , 50239276.966254, 50238694.377885, 50241258.305094, 50237790.288386, 50240320.464294, 50239819.00547 , 50238272.447008, 50242340.252394, 50235733.502502, 50240781.350132, 50242820.452884, 50243260.115676, 50241819.533841, 50238071.211777, 50240656.570545, 50234092.084342, 50234494.794375, 50234856.734131, 50236618.428003, 50238714.003412, 50236196.295122, 50239114.916644, 50236999.909492, 50241201.685427, 50248229.369524, 50245735.704121, 50246294.791182, 50248767.166384, 50243211.426168, 50243791.784211, 50244331.724518, 50244831.158016, 50246813.437152, 50247291.676182, 50245290.154576, 50246086.730154, 50246424.316582, 50244017.9306 , 50245708.66943 , 50243659.302194, 50244336.072468, 50237340.695538, 50241920.64028 , 50241581.431299, 50239475.199931, 50247729.493096])
- phys_cell_id(cell)int32...
- long_name :
- physical domain ID of cell
- grid_type :
- unstructured
- number_of_grid_in_reference :
- 1
array([1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1], dtype=int32)
- phys_edge_id(edge)int32...
- long_name :
- physical domain ID of edge
array([1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1], dtype=int32)
- lon_cell_centre(cell)float64...
- long_name :
- longitude of cell centre
- units :
- radian
- grid_type :
- unstructured
- number_of_grid_in_reference :
- 1
array([0.180381, 0.180509, 0.179222, 0.178862, 0.178989, 0.177701, 0.17975 , 0.181041, 0.180912, 0.180152, 0.176936, 0.178098, 0.176043, 0.17681 , 0.178463, 0.177575, 0.178336, 0.179622, 0.179979, 0.181136, 0.179096, 0.179518, 0.178625, 0.17939 , 0.180686, 0.179921, 0.180815, 0.18005 , 0.178752, 0.177332, 0.177458, 0.176168, 0.178225, 0.180281, 0.173859, 0.172969, 0.1759 , 0.177054, 0.175012, 0.174369, 0.175526, 0.173477, 0.174246, 0.176291, 0.175136, 0.177179, 0.176416, 0.174758, 0.17565 , 0.174882, 0.1736 , 0.173091, 0.17794 , 0.173214, 0.174111, 0.173337, 0.17256 , 0.175791, 0.174625, 0.176689, 0.173725, 0.175398, 0.174501, 0.175273, 0.176563, 0.177984, 0.177857, 0.172828, 0.172705, 0.173987])
- lat_cell_centre(cell)float64...
- long_name :
- latitude of cell centre
- units :
- radian
- grid_type :
- unstructured
- number_of_grid_in_reference :
- 1
array([0.945285, 0.945958, 0.945008, 0.947452, 0.948124, 0.947173, 0.947041, 0.947991, 0.947319, 0.948402, 0.948256, 0.948535, 0.948666, 0.947584, 0.946091, 0.946501, 0.945418, 0.946369, 0.943924, 0.944201, 0.944334, 0.950157, 0.950568, 0.949486, 0.950435, 0.951518, 0.951106, 0.952188, 0.951239, 0.949618, 0.950289, 0.949338, 0.949207, 0.949074, 0.944315, 0.944723, 0.944186, 0.944466, 0.944595, 0.946351, 0.946631, 0.94676 , 0.945678, 0.945548, 0.945269, 0.945139, 0.946221, 0.947713, 0.947304, 0.948385, 0.947432, 0.945397, 0.944056, 0.949877, 0.949467, 0.950548, 0.951629, 0.951782, 0.951501, 0.951371, 0.951911, 0.950419, 0.95083 , 0.949748, 0.9507 , 0.952321, 0.951651, 0.948514, 0.947841, 0.948795])
- lat_cell_barycenter(cell)float64...
- long_name :
- latitude of cell barycenter
- units :
- radian
- grid_type :
- unstructured
- number_of_grid_in_reference :
- 1
array([0.945283, 0.945961, 0.94501 , 0.947449, 0.948127, 0.947176, 0.947044, 0.947994, 0.947316, 0.948399, 0.948259, 0.948532, 0.948663, 0.947581, 0.946093, 0.946498, 0.945415, 0.946366, 0.943927, 0.944199, 0.944332, 0.95016 , 0.950565, 0.949482, 0.950432, 0.951514, 0.951109, 0.952192, 0.951242, 0.949614, 0.950292, 0.949341, 0.94921 , 0.949077, 0.944317, 0.94472 , 0.944189, 0.944463, 0.944593, 0.946353, 0.946628, 0.946757, 0.945675, 0.945546, 0.945271, 0.945142, 0.946224, 0.94771 , 0.947307, 0.948389, 0.947435, 0.945399, 0.944058, 0.949873, 0.94947 , 0.950551, 0.951632, 0.951778, 0.951504, 0.951374, 0.951907, 0.950423, 0.950826, 0.949745, 0.950696, 0.952324, 0.951647, 0.948517, 0.947838, 0.948792])
- lon_cell_barycenter(cell)float64...
- long_name :
- longitude of cell barycenter
- units :
- radian
- grid_type :
- unstructured
- number_of_grid_in_reference :
- 1
array([0.180356, 0.180533, 0.179247, 0.178837, 0.179013, 0.177725, 0.179774, 0.181066, 0.180888, 0.180128, 0.17696 , 0.178074, 0.176019, 0.176786, 0.178487, 0.177551, 0.178312, 0.179598, 0.180004, 0.181112, 0.179071, 0.179542, 0.1786 , 0.179366, 0.180661, 0.179897, 0.180839, 0.180075, 0.178777, 0.177308, 0.177482, 0.176192, 0.178249, 0.180305, 0.173883, 0.172946, 0.175924, 0.17703 , 0.174989, 0.174393, 0.175502, 0.173453, 0.174222, 0.176268, 0.175159, 0.177203, 0.17644 , 0.174734, 0.175674, 0.174906, 0.173623, 0.173115, 0.177964, 0.17319 , 0.174134, 0.17336 , 0.172584, 0.175768, 0.174649, 0.176713, 0.173702, 0.175422, 0.174477, 0.175249, 0.176539, 0.178008, 0.177833, 0.172851, 0.172682, 0.173964])
- longitude_vertices(vertex)float64...
- long_name :
- longitude of vertices
- units :
- radian
array([0.181467, 0.18071 , 0.182221, 0.182002, 0.179422, 0.18018 , 0.178138, 0.179951, 0.177899, 0.178662, 0.179189, 0.176613, 0.181244, 0.177134, 0.175846, 0.175077, 0.177377, 0.180935, 0.178897, 0.181781, 0.181018, 0.180253, 0.179719, 0.178425, 0.180483, 0.177657, 0.178953, 0.176365, 0.172778, 0.174818, 0.174052, 0.172008, 0.176858, 0.176095, 0.175331, 0.174563, 0.173284, 0.172513, 0.173793, 0.172245, 0.171467, 0.173531, 0.17302 , 0.174305, 0.172754, 0.178184, 0.174821, 0.175594, 0.176887, 0.171739])
- latitude_vertices(vertex)float64...
- long_name :
- latitude of vertices
- units :
- radian
array([0.945555, 0.946639, 0.944471, 0.947588, 0.945688, 0.944605, 0.944737, 0.947722, 0.947854, 0.946771, 0.948805, 0.946902, 0.948671, 0.948937, 0.947985, 0.949067, 0.94582 , 0.943521, 0.943653, 0.950703, 0.951786, 0.952869, 0.950837, 0.949887, 0.949754, 0.95097 , 0.95192 , 0.950019, 0.944041, 0.943914, 0.944996, 0.945123, 0.943784, 0.944867, 0.94595 , 0.947032, 0.946078, 0.94716 , 0.948114, 0.950276, 0.951357, 0.951229, 0.949195, 0.950148, 0.95231 , 0.953002, 0.952182, 0.9511 , 0.952052, 0.948241])
- lon_edge_centre(edge)float64...
- long_name :
- longitudes of edge midpoints
- units :
- radian
array([0.181089, 0.181356, 0.180445, 0.179801, 0.180823, 0.180066, 0.17878 , 0.179159, 0.181201, 0.178925, 0.178281, 0.179306, 0.17957 , 0.178544, 0.177256, 0.177638, 0.180331, 0.179686, 0.180977, 0.181623, 0.180597, 0.180217, 0.177517, 0.17649 , 0.176873, 0.178161, 0.176106, 0.175462, 0.17623 , 0.178019, 0.1784 , 0.179042, 0.176995, 0.177758, 0.181578, 0.180558, 0.179538, 0.179916, 0.178518, 0.180636, 0.1814 , 0.179072, 0.179454, 0.180102, 0.178688, 0.178041, 0.178807, 0.179836, 0.18075 , 0.181132, 0.180368, 0.179986, 0.179337, 0.179603, 0.178305, 0.177395, 0.17675 , 0.177779, 0.177011, 0.175721, 0.180864, 0.173798, 0.173415, 0.174435, 0.172393, 0.17303 , 0.175838, 0.176477, 0.175456, 0.177497, 0.177117, 0.175074, 0.174947, 0.173923, 0.174307, 0.175971, 0.175588, 0.173538, 0.172899, 0.174691, 0.173668, 0.175713, 0.176736, 0.176354, 0.175204, 0.17482 , 0.174179, 0.174435, 0.173153, 0.172646, 0.177877, 0.172888, 0.172499, 0.172633, 0.173663, 0.173275, 0.174049, 0.174692, 0.173919, 0.17211 , 0.173143, 0.175208, 0.17624 , 0.175854, 0.174176, 0.174563, 0.176626, 0.177273, 0.173787, 0.17495 , 0.175336, 0.17598 , 0.17792 , 0.178569, 0.177535, 0.172126, 0.172766, 0.173407, 0.172379])
- lat_edge_centre(edge)float64...
- long_name :
- latitudes of edge midpoints
- units :
- radian
array([0.946097, 0.947113, 0.945622, 0.945146, 0.94508 , 0.946163, 0.945213, 0.944671, 0.944538, 0.947788, 0.947313, 0.947247, 0.948263, 0.94833 , 0.947378, 0.946837, 0.94718 , 0.946705, 0.947655, 0.94813 , 0.948197, 0.948738, 0.948395, 0.948461, 0.94792 , 0.948871, 0.949002, 0.948526, 0.947444, 0.946296, 0.945754, 0.94623 , 0.946361, 0.945278, 0.943996, 0.944063, 0.944129, 0.943587, 0.944195, 0.952327, 0.951245, 0.950362, 0.949821, 0.950296, 0.950904, 0.950429, 0.949346, 0.94928 , 0.95077 , 0.950229, 0.951312, 0.951853, 0.951378, 0.952394, 0.951445, 0.949953, 0.949478, 0.949412, 0.950494, 0.949543, 0.949213, 0.943978, 0.944519, 0.944455, 0.944582, 0.94506 , 0.943849, 0.944326, 0.944391, 0.944261, 0.944802, 0.944932, 0.946491, 0.946555, 0.946014, 0.946426, 0.946967, 0.947096, 0.946619, 0.945473, 0.945537, 0.945409, 0.945344, 0.945885, 0.947508, 0.948049, 0.947573, 0.94859 , 0.947637, 0.945601, 0.943719, 0.950753, 0.951293, 0.949736, 0.949672, 0.950212, 0.949131, 0.949607, 0.950689, 0.951834, 0.95177 , 0.951641, 0.951576, 0.952117, 0.951706, 0.951165, 0.951035, 0.951511, 0.952246, 0.950624, 0.950084, 0.95056 , 0.951986, 0.952461, 0.952527, 0.9477 , 0.948178, 0.948654, 0.948718])
- edge_of_cell(nv, cell)int32...
- long_name :
- edges of each cellvertices
array([[ 3, 1, 7, ..., 117, 116, 88], [ 4, 6, 8, ..., 118, 89, 97], [ 5, 3, 4, ..., 119, 117, 118]], dtype=int32)
- vertex_of_cell(nv, cell)int32...
- long_name :
- vertices of each cellcells ad
array([[ 5, 1, 5, ..., 39, 50, 39], [ 6, 2, 7, ..., 43, 38, 16], [ 1, 5, 6, ..., 50, 39, 43]], dtype=int32)
- adjacent_cell_of_edge(nc, edge)int32...
- long_name :
- cells adjacent to each edge
array([[-1, -1, 1, ..., 68, 68, 68], [ 2, 9, 2, ..., 69, 70, -1]], dtype=int32)
- edge_vertices(nc, edge)int32...
- long_name :
- vertices at the end of of each edge
array([[ 2, 4, 1, ..., 50, 39, 43], [ 1, 2, 5, ..., 39, 43, 50]], dtype=int32)
- cells_of_vertex(ne, vertex)int32...
- long_name :
- cells around each vertex
array([[-1, -1, -1, ..., 58, 58, -1], [-1, -1, -1, ..., 59, 60, -1], [-1, 2, -1, ..., 60, -1, -1], [ 1, 7, -1, ..., 62, 66, 68], [ 2, 9, 20, ..., 63, 67, 69], [-1, 18, -1, ..., 65, -1, -1]], dtype=int32)
- edges_of_vertex(ne, vertex)int32...
- long_name :
- edges around each vertex
array([[ -1, -1, -1, ..., 102, 103, -1], [ -1, 1, -1, ..., 103, 104, -1], [ 1, 2, 9, ..., 106, 108, 116], [ -1, 6, -1, ..., 107, -1, -1], [ 3, 17, 35, ..., 110, 113, 117], [ 5, 18, -1, ..., 112, 115, 119]], dtype=int32)
- vertices_of_vertex(ne, vertex)int32...
- long_name :
- vertices around each vertex
array([[-1, -1, 1, ..., 47, 48, -1], [-1, 1, -1, ..., 49, 47, -1], [ 2, 4, 6, ..., 42, 26, 38], [ 3, 5, -1, ..., 26, -1, -1], [ 5, 8, 18, ..., 44, 27, 39], [ 6, 10, -1, ..., 28, 46, 43]], dtype=int32)
- cell_area_p(cell)float64...
- long_name :
- area of grid cell
- units :
- m2
- grid_type :
- unstructured
- number_of_grid_in_reference :
- 1
array([25122696.100735, 25115307.267907, 25116300.534128, 25123237.931024, 25115830.247435, 25116842.75659 , 25115578.910138, 25114562.040095, 25121960.932241, 25122212.059399, 25117083.448929, 25123478.41463 , 25124729.658542, 25124499.793522, 25116581.784901, 25124249.665799, 25123979.275508, 25122977.159648, 25115999.004667, 25122394.754638, 25123688.622955, 25115025.063522, 25122653.375085, 25122442.873669, 25121393.215094, 25121593.997027, 25113974.845723, 25114166.124292, 25115226.0792 , 25123698.610424, 25116272.006796, 25117303.861822, 25116061.279655, 25114803.717182, 25119795.715901, 25127478.523255, 25118545.371572, 25124967.236121, 25126230.550547, 25119085.469371, 25125506.966573, 25126749.018405, 25126499.890636, 25125247.219992, 25118825.534301, 25117570.619972, 25117841.200154, 25125746.475592, 25118091.526784, 25118321.599695, 25119325.176469, 25120065.233457, 25117279.786595, 25126164.780769, 25118531.418818, 25118720.984305, 25118890.296435, 25124078.139111, 25117683.849702, 25116462.429012, 25125297.678096, 25117503.995341, 25125128.600582, 25124939.260832, 25123898.518499, 25115406.764477, 25122843.563845, 25119544.655465, 25126977.933665, 25125965.746968])
- cell_elevation(cell)float64...
- long_name :
- elevation at the cell centers
- units :
- m
- grid_type :
- unstructured
- number_of_grid_in_reference :
- 1
array([ 33., 30., 47., 18., 27., 35., 23., 44., 37., 16., 0., 33., 23., 39., 20., 33., 33., 0., 54., 40., 35., -20., -20., 4., -20., -20., -20., -20., -20., 17., 0., 25., 0., 18., 22., 21., 33., 52., 28., 42., 33., 38., 35., 30., 22., 42., 63., 8., 22., 25., 0., 24., 50., 20., 20., 0., 21., -22., 0., -20., 24., 31., 0., 21., 33., -20., -20., 10., 14., 16.])
- cell_sea_land_mask(cell)int32...
- long_name :
- sea (-2 inner, -1 boundary) land (2 inner, 1 boundary) mask for the cell
- units :
- 2,1,-1,-
- grid_type :
- unstructured
- number_of_grid_in_reference :
- 1
array([ 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, -1, -1, 1, -2, -2, -2, -2, -2, 2, 1, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, -1, 1, -1, 2, 2, 2, 2, 1, -2, -2, 2, 2, 2], dtype=int32)
- cell_domain_id(cell, max_stored_decompositions)int32...
- long_name :
- cell domain id for decomposition
array([[-1, -1, -1, -1], [-1, -1, -1, -1], [-1, -1, -1, -1], ..., [-1, -1, -1, -1], [-1, -1, -1, -1], [-1, -1, -1, -1]], dtype=int32)
- cell_no_of_domains(max_stored_decompositions)int32...
- long_name :
- number of domains for each decomposition
array([0, 0, 0, 0], dtype=int32)
- dual_area_p(vertex)float64...
- long_name :
- areas of dual hexagonal/pentagonal cells
- units :
- m2
array([50236704.122656, 50237267.527659, 50236100.118676, 50235230.038 , 50239276.966254, 50238694.377885, 50241258.305094, 50237790.288386, 50240320.464294, 50239819.00547 , 50238272.447008, 50242340.252394, 50235733.502502, 50240781.350132, 50242820.452884, 50243260.115676, 50241819.533841, 50238071.211777, 50240656.570545, 50234092.084342, 50234494.794375, 50234856.734131, 50236618.428003, 50238714.003412, 50236196.295122, 50239114.916644, 50236999.909492, 50241201.685427, 50248229.369524, 50245735.704121, 50246294.791182, 50248767.166384, 50243211.426168, 50243791.784211, 50244331.724518, 50244831.158016, 50246813.437152, 50247291.676182, 50245290.154576, 50246086.730154, 50246424.316582, 50244017.9306 , 50245708.66943 , 50243659.302194, 50244336.072468, 50237340.695538, 50241920.64028 , 50241581.431299, 50239475.199931, 50247729.493096])
- edge_length(edge)float64...
- long_name :
- lengths of edges of triangular cells
- units :
- m
array([7457.780512, 7726.180508, 7671.406511, 7459.621232, 7727.687566, 7726.220633, 7726.260814, 7669.834808, 7669.853271, 7674.502786, 7459.646004, 7724.750261, 7457.805155, 7723.27646 , 7723.314953, 7672.939478, 7457.793181, 7672.956351, 7674.51952 , 7455.952435, 7724.710996, 7676.06173 , 7459.657368, 7721.836883, 7674.486711, 7676.04581 , 7676.030534, 7461.509045, 7461.49828 , 7724.789599, 7671.388842, 7459.633959, 7461.486846, 7461.474739, 7729.151052, 7459.607825, 7727.728586, 7668.277383, 7461.461964, 7454.144998, 7454.134638, 7721.761694, 7677.60052 , 7455.975515, 7679.135885, 7457.827018, 7457.816435, 7723.238056, 7679.150804, 7723.199755, 7721.724273, 7680.681903, 7455.985991, 7720.245381, 7720.28192 , 7677.585415, 7459.668047, 7721.799235, 7720.318597, 7720.355397, 7455.964329, 7668.223742, 7723.430818, 7465.168374, 7467.020586, 7669.783457, 7668.240953, 7463.31549 , 7724.868432, 7726.301033, 7669.81703 , 7669.799919, 7463.339143, 7721.912434, 7671.355497, 7723.35352 , 7672.923261, 7672.907682, 7465.190823, 7723.392146, 7465.179922, 7463.327646, 7724.828994, 7671.371843, 7721.87462 , 7674.471276, 7463.349981, 7720.392303, 7720.429302, 7721.950308, 7668.258827, 7717.417451, 7679.09484 , 7463.36967 , 7718.906577, 7677.557073, 7676.015883, 7461.519135, 7461.528551, 7715.924931, 7461.537292, 7459.687348, 7718.834554, 7680.654345, 7717.382211, 7679.107921, 7679.121596, 7457.836905, 7680.641471, 7718.870503, 7677.570938, 7459.678041, 7680.667816, 7455.99576 , 7718.798742, 7465.201074, 7674.456465, 7463.360156, 7718.94276 ])
- edge_cell_distance(nc, edge)float64...
- long_name :
- distances between edge midpoint and adjacent triangle midpoints
- units :
- m
array([[2336.803793, 2106.251189, 2157.070769, ..., 2153.383914, 2331.511663, 2113.258962], [2335.686969, 2107.583409, 2155.791416, ..., 2154.672351, 2332.6356 , 2114.587976]])
- dual_edge_length(edge)float64...
- long_name :
- lengths of dual edges (distances between triangular cell circumcenters)
- units :
- m
array([4672.490762, 4213.834599, 4312.862184, 4669.678136, 4211.161948, 4213.90321 , 4213.969957, 4315.792787, 4315.572287, 4307.439926, 4669.723156, 4216.643892, 4672.532448, 4219.383993, 4219.457665, 4310.364197, 4672.513121, 4310.151397, 4307.2274 , 4675.320017, 4216.571785, 4304.519134, 4669.741108, 4222.200643, 4307.648891, 4304.727786, 4304.932928, 4666.946029, 4666.929453, 4216.71409 , 4313.078834, 4669.702163, 4666.909838, 4666.887184, 4208.420129, 4669.651066, 4211.225256, 4318.506047, 4666.861483, 4678.161755, 4678.151791, 4222.044314, 4301.810204, 4675.352322, 4299.100626, 4672.562013, 4672.548744, 4219.30836 , 4298.896203, 4219.230736, 4221.963106, 4296.189877, 4675.363935, 4224.694813, 4224.779626, 4302.015003, 4669.75603 , 4222.123483, 4224.862345, 4224.943008, 4675.337682, 4319.157088, 4219.66719 , 4661.271327, 4658.470828, 4316.432483, 4318.943715, 4664.06823 , 4216.848863, 4214.034862, 4316.009632, 4316.222851, 4664.113824, 4222.349043, 4313.501314, 4219.529402, 4310.57342 , 4310.779107, 4661.314171, 4219.599234, 4661.29427 , 4664.092545, 4216.782409, 4313.291866, 4222.275817, 4307.854322, 4664.132062, 4225.021651, 4225.098281, 4222.420343, 4318.726717, 4230.511466, 4299.693227, 4664.15942 , 4227.766868, 4302.414208, 4305.13458 , 4666.959572, 4666.970082, 4233.255425, 4666.97756 , 4669.776774, 4227.600568, 4296.587531, 4230.425862, 4299.499097, 4299.301575, 4672.572245, 4296.781268, 4227.684754, 4302.216325, 4669.767918, 4296.390412, 4675.372524, 4227.51428 , 4661.331021, 4308.056265, 4664.147263, 4227.846938])
- edgequad_area(edge)float64...
- long_name :
- area around the edge formed by the two adjacent triangles
- units :
- m2
array([4.292217e-07, 4.010199e-07, 4.075343e-07, 4.290692e-07, 4.008437e-07, 4.010285e-07, 4.010369e-07, 4.077277e-07, 4.077078e-07, 4.071862e-07, 4.290747e-07, 4.012130e-07, 4.292269e-07, 4.013971e-07, 4.014061e-07, 4.073796e-07, 4.292244e-07, 4.073604e-07, 4.071670e-07, 4.293763e-07, 4.012041e-07, 4.069928e-07, 4.290770e-07, 4.015902e-07, 4.072051e-07, 4.070116e-07, 4.070302e-07, 4.289266e-07, 4.289245e-07, 4.012217e-07, 4.075538e-07, 4.290721e-07, 4.289220e-07, 4.289193e-07, 4.006586e-07, 4.290659e-07, 4.008519e-07, 4.079011e-07, 4.289162e-07, 4.295331e-07, 4.295316e-07, 4.015714e-07, 4.068182e-07, 4.293806e-07, 4.066432e-07, 4.292309e-07, 4.292290e-07, 4.013879e-07, 4.066247e-07, 4.013785e-07, 4.015617e-07, 4.064497e-07, 4.293822e-07, 4.017446e-07, 4.017545e-07, 4.068367e-07, 4.290790e-07, 4.015809e-07, 4.017643e-07, 4.017739e-07, 4.293786e-07, 4.079598e-07, 4.014320e-07, 4.286152e-07, 4.284640e-07, 4.077854e-07, 4.079405e-07, 4.287659e-07, 4.012386e-07, 4.010452e-07, 4.077472e-07, 4.077664e-07, 4.287715e-07, 4.016082e-07, 4.075920e-07, 4.014149e-07, 4.073985e-07, 4.074172e-07, 4.286204e-07, 4.014236e-07, 4.286180e-07, 4.287689e-07, 4.012302e-07, 4.075730e-07, 4.015993e-07, 4.072237e-07, 4.287738e-07, 4.017833e-07, 4.017925e-07, 4.016170e-07, 4.079210e-07, 4.021503e-07, 4.066971e-07, 4.287774e-07, 4.019670e-07, 4.068730e-07, 4.070485e-07, 4.289285e-07, 4.289300e-07, 4.023334e-07, 4.289312e-07, 4.290820e-07, 4.019474e-07, 4.064859e-07, 4.021404e-07, 4.066794e-07, 4.066615e-07, 4.292324e-07, 4.065035e-07, 4.019573e-07, 4.068550e-07, 4.290807e-07, 4.064679e-07, 4.293836e-07, 4.019374e-07, 4.286226e-07, 4.072420e-07, 4.287758e-07, 4.019765e-07])
- edge_elevation(edge)float64...
- long_name :
- elevation at the edge centers
- units :
- m
array([ 38., 58., 33., 47., 33., 30., 47., 47., 40., 27., 35., 23., 27., 33., 39., 35., 37., 23., 44., 44., 44., 18., 33., 23., 39., 33., 25., 25., 39., 33., 33., 20., 63., 42., 40., 54., 54., 54., 50., -20., -20., -20., 0., -20., -20., 0., 4., 18., -20., -20., -20., -20., -20., -20., -20., 17., 25., 17., 33., 25., 18., 22., 22., 28., 21., 24., 33., 52., 33., 52., 52., 28., 42., 42., 42., 63., 33., 38., 38., 35., 35., 30., 42., 63., 22., 25., 8., 25., 14., 24., 50., 0., 21., 28., 20., 20., 20., 21., 0., 31., 24., 0., -20., -20., 24., 0., 0., -20., 24., 31., 31., 33., -20., -20., -20., 17., 14., 16., 13.])
- edge_sea_land_mask(edge)int32...
- long_name :
- sea (-2 inner, -1 boundary) land (2 inner, 1 boundary) mask for the cell
- units :
- 2,1,-1,-
array([ 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, -2, -2, -2, 0, -2, -2, 0, 2, 2, -2, -2, -2, -2, -2, -2, -2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 0, -2, -2, 2, 2, 0, -2, 2, 2, 2, 2, -2, -2, -2, 2, 2, 2, 2], dtype=int32)
- edge_vert_distance(nc, edge)float64...
- long_name :
- distances between edge midpoint and vertices of that edge
- units :
- m
array([[3728.890256, 3863.090254, 3835.703256, ..., 3837.228233, 3731.680078, 3859.47138 ], [3728.890256, 3863.090254, 3835.703256, ..., 3837.228233, 3731.680078, 3859.47138 ]])
- zonal_normal_primal_edge(edge)float64...
- long_name :
- zonal component of normal to primal edge
- units :
- radian
array([-0.925783, -0.782843, 0.11073 , -0.925421, 0.783396, -0.783674, -0.784501, -0.109785, -0.111175, 0.109832, -0.924809, 0.783953, 0.925174, -0.784235, -0.785062, -0.108886, 0.925479, -0.110282, -0.111232, 0.925843, -0.783123, 0.110782, 0.924501, -0.785344, -0.108432, 0.10938 , 0.107977, -0.923825, -0.924136, -0.784781, -0.109336, 0.925116, -0.924446, -0.924753, -0.783119, 0.925724, -0.784224, -0.11023 , -0.925059, -0.925293, -0.9256 , -0.783687, -0.11033 , 0.925233, 0.109876, -0.924558, -0.924867, 0.783404, 0.111285, 0.78257 , 0.782853, 0.110831, -0.924925, -0.783138, -0.783971, 0.108924, -0.924191, 0.784517, -0.784802, -0.785628, -0.925539, 0.106068, -0.787522, 0.92372 , 0.923045, 0.105612, -0.107455, 0.924391, -0.786425, 0.785325, 0.108394, 0.107003, 0.923772, -0.786988, -0.106549, 0.785885, 0.107489, 0.106092, -0.923095, 0.786706, -0.923408, -0.924082, 0.785605, 0.107943, 0.786168, 0.107032, -0.92346 , -0.786452, -0.787271, 0.787804, 0.108843, 0.787024, 0.105648, -0.922831, 0.786737, 0.106112, -0.106573, 0.923513, 0.923198, 0.787313, 0.922882, -0.923567, 0.785087, 0.108006, -0.786201, -0.107057, -0.108467, 0.924248, -0.106594, -0.785914, -0.107518, 0.92388 , -0.109419, 0.924616, -0.784257, 0.92278 , -0.105632, 0.923146, -0.787557])
- meridional_normal_primal_edge(edge)float64...
- long_name :
- meridional component of normal to primal edge
- units :
- radian
array([-0.378055, 0.622219, 0.993851, -0.378941, -0.621523, 0.621173, 0.620127, -0.993955, -0.993801, 0.99395 , -0.380431, -0.62082 , 0.379543, 0.620465, 0.619418, -0.994054, 0.378798, -0.9939 , -0.993795, 0.377907, 0.621867, 0.993845, 0.381179, 0.619059, -0.994104, 0.994 , 0.994153, -0.382814, -0.382063, 0.619774, -0.994005, 0.379685, -0.381314, -0.380567, 0.621871, 0.378199, 0.620478, -0.993906, -0.379823, -0.379252, -0.378504, 0.621156, -0.993895, 0.379399, 0.993945, -0.381041, -0.380291, -0.621513, 0.993789, -0.622563, -0.622207, 0.993839, -0.380149, 0.621848, 0.620797, 0.99405 , -0.38193 , -0.620107, 0.619747, 0.618699, -0.378652, 0.994359, 0.616286, 0.383069, 0.384691, 0.994407, -0.99421 , 0.381446, 0.617686, -0.619083, 0.994108, 0.994259, 0.382943, 0.616969, -0.994307, -0.618372, 0.994206, 0.994356, -0.384572, -0.617328, -0.383819, -0.382193, -0.618729, 0.994157, -0.618013, 0.994256, -0.383695, 0.617652, 0.616607, -0.615926, 0.994059, -0.616922, 0.994404, -0.385206, -0.617288, 0.994354, -0.994305, 0.383568, 0.384324, -0.616554, 0.385082, -0.383438, -0.619385, 0.99415 , 0.617971, -0.994253, -0.9941 , 0.381794, -0.994303, 0.618336, -0.994203, 0.382683, -0.993996, 0.3809 , 0.620436, 0.385326, -0.994405, 0.384449, 0.616242])
- zonal_normal_dual_edge(edge)float64...
- long_name :
- zonal component of normal to dual edge
- units :
- radian
array([-0.378055, 0.622219, 0.993851, -0.378941, -0.621523, 0.621173, 0.620127, -0.993955, -0.993801, 0.99395 , -0.380431, -0.62082 , 0.379543, 0.620465, 0.619418, -0.994054, 0.378798, -0.9939 , -0.993795, 0.377907, 0.621867, 0.993845, 0.381179, 0.619059, -0.994104, 0.994 , 0.994153, -0.382814, -0.382063, 0.619774, -0.994005, 0.379685, -0.381314, -0.380567, 0.621871, 0.378199, 0.620478, -0.993906, -0.379823, -0.379252, -0.378504, 0.621156, -0.993895, 0.379399, 0.993945, -0.381041, -0.380291, -0.621513, 0.993789, -0.622563, -0.622207, 0.993839, -0.380149, 0.621848, 0.620797, 0.99405 , -0.38193 , -0.620107, 0.619747, 0.618699, -0.378652, 0.994359, 0.616286, 0.383069, 0.384691, 0.994407, -0.99421 , 0.381446, 0.617686, -0.619083, 0.994108, 0.994259, 0.382943, 0.616969, -0.994307, -0.618372, 0.994206, 0.994356, -0.384572, -0.617328, -0.383819, -0.382193, -0.618729, 0.994157, -0.618013, 0.994256, -0.383695, 0.617652, 0.616607, -0.615926, 0.994059, -0.616922, 0.994404, -0.385206, -0.617288, 0.994354, -0.994305, 0.383568, 0.384324, -0.616554, 0.385082, -0.383438, -0.619385, 0.99415 , 0.617971, -0.994253, -0.9941 , 0.381794, -0.994303, 0.618336, -0.994203, 0.382683, -0.993996, 0.3809 , 0.620436, 0.385326, -0.994405, 0.384449, 0.616242])
- meridional_normal_dual_edge(edge)float64...
- long_name :
- meridional component of normal to dual edge
- units :
- radian
array([ 0.925783, 0.782843, -0.11073 , 0.925421, -0.783396, 0.783674, 0.784501, 0.109785, 0.111175, -0.109832, 0.924809, -0.783953, -0.925174, 0.784235, 0.785062, 0.108886, -0.925479, 0.110282, 0.111232, -0.925843, 0.783123, -0.110782, -0.924501, 0.785344, 0.108432, -0.10938 , -0.107977, 0.923825, 0.924136, 0.784781, 0.109336, -0.925116, 0.924446, 0.924753, 0.783119, -0.925724, 0.784224, 0.11023 , 0.925059, 0.925293, 0.9256 , 0.783687, 0.11033 , -0.925233, -0.109876, 0.924558, 0.924867, -0.783404, -0.111285, -0.78257 , -0.782853, -0.110831, 0.924925, 0.783138, 0.783971, -0.108924, 0.924191, -0.784517, 0.784802, 0.785628, 0.925539, -0.106068, 0.787522, -0.92372 , -0.923045, -0.105612, 0.107455, -0.924391, 0.786425, -0.785325, -0.108394, -0.107003, -0.923772, 0.786988, 0.106549, -0.785885, -0.107489, -0.106092, 0.923095, -0.786706, 0.923408, 0.924082, -0.785605, -0.107943, -0.786168, -0.107032, 0.92346 , 0.786452, 0.787271, -0.787804, -0.108843, -0.787024, -0.105648, 0.922831, -0.786737, -0.106112, 0.106573, -0.923513, -0.923198, -0.787313, -0.922882, 0.923567, -0.785087, -0.108006, 0.786201, 0.107057, 0.108467, -0.924248, 0.106594, 0.785914, 0.107518, -0.92388 , 0.109419, -0.924616, 0.784257, -0.92278 , 0.105632, -0.923146, 0.787557])
- orientation_of_normal(nv, cell)int32...
- long_name :
- orientations of normals to triangular cell edges
array([[ 1, -1, 1, ..., 1, -1, -1], [ 1, 1, 1, ..., 1, -1, -1], [ 1, -1, -1, ..., 1, -1, -1]], dtype=int32)
- cell_index(cell)int32...
- long_name :
- cell index
array([ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70], dtype=int32)
- parent_cell_index(cell)int32...
- long_name :
- parent cell index
array([1070595, 1070595, 1070595, 1070601, 1070601, 1070601, 1070601, 1070602, 1070602, 1070602, 1070603, 1070603, 1070603, 1070603, 1070604, 1070604, 1070604, 1070604, 1070606, 1070606, 1070606, 1070621, 1070621, 1070621, 1070621, 1070622, 1070622, 1070622, 1070622, 1070623, 1070623, 1070623, 1070623, 1070624, 1070625, 1070626, 1070628, 1070628, 1070628, 1070629, 1070629, 1070629, 1070629, 1070630, 1070630, 1070630, 1070630, 1070631, 1070631, 1070631, 1070631, 1070632, 1070640, 1070735, 1070735, 1070735, 1070736, 1070741, 1070741, 1070741, 1070742, 1070743, 1070743, 1070743, 1070743, 1070744, 1070744, 1070783, 1070783, 1070783], dtype=int32)
- parent_cell_type(cell)int32...
- long_name :
- parent cell type
array([200, 203, 201, 200, 201, 202, 203, 200, 203, 202, 200, 203, 201, 202, 200, 203, 201, 202, 200, 203, 201, 200, 201, 202, 203, 200, 203, 201, 202, 200, 203, 201, 202, 203, 203, 203, 200, 201, 202, 200, 201, 202, 203, 200, 203, 201, 202, 200, 203, 201, 202, 202, 201, 200, 201, 202, 203, 200, 201, 202, 202, 200, 203, 201, 202, 200, 203, 200, 203, 201], dtype=int32)
- neighbor_cell_index(nv, cell)int32...
- long_name :
- cell neighbor index
array([[ 2, -1, 17, ..., 69, -1, 50], [ 3, 18, 21, ..., 70, 51, 55], [-1, 1, 1, ..., -1, 68, 68]], dtype=int32)
- child_cell_index(no, cell)int32...
- long_name :
- child cell index
array([[0, 0, 0, ..., 0, 0, 0], [0, 0, 0, ..., 0, 0, 0], [0, 0, 0, ..., 0, 0, 0], [0, 0, 0, ..., 0, 0, 0]], dtype=int32)
- child_cell_id(cell)int32...
- long_name :
- domain ID of child cell
array([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0], dtype=int32)
- edge_index(edge)int32...
- long_name :
- edge index
array([6427303, 6427312, 6427313, 6427314, 6427315, 6427316, 6427317, 6427318, 6427319, 6427353, 6427354, 6427355, 6427356, 6427357, 6427358, 6427359, 6427360, 6427361, 6427362, 6427363, 6427364, 6427366, 6427367, 6427368, 6427369, 6427370, 6427371, 6427372, 6427373, 6427374, 6427375, 6427376, 6427377, 6427378, 6427386, 6427388, 6427389, 6427390, 6427391, 6427426, 6427427, 6427483, 6427484, 6427485, 6427486, 6427487, 6427488, 6427489, 6427490, 6427491, 6427492, 6427493, 6427494, 6427495, 6427496, 6427497, 6427498, 6427499, 6427500, 6427501, 6427502, 6427505, 6427508, 6427509, 6427514, 6427517, 6427528, 6427529, 6427530, 6427532, 6427533, 6427534, 6427535, 6427536, 6427537, 6427538, 6427539, 6427540, 6427541, 6427542, 6427543, 6427544, 6427545, 6427546, 6427547, 6427548, 6427549, 6427550, 6427551, 6427554, 6427603, 6428152, 6428153, 6428161, 6428162, 6428163, 6428165, 6428166, 6428167, 6428168, 6428171, 6428203, 6428204, 6428205, 6428206, 6428207, 6428208, 6428209, 6428214, 6428215, 6428216, 6428217, 6428218, 6428219, 6428220, 6428411, 6428419, 6428420, 6428421], dtype=int32)
- edge_parent_type(edge)int32...
- long_name :
- edge paren
array([101, 102, 202, 203, 201, 101, 102, 101, 102, 203, 201, 202, 102, 101, 102, 101, 101, 102, 202, 203, 201, 102, 202, 203, 201, 101, 102, 101, 102, 202, 203, 201, 101, 102, 101, 202, 203, 201, 101, 101, 102, 203, 201, 202, 102, 101, 102, 101, 101, 102, 202, 203, 201, 101, 102, 202, 203, 201, 101, 102, 202, 202, 101, 102, 202, 101, 202, 203, 201, 102, 101, 102, 203, 201, 202, 102, 101, 102, 101, 101, 102, 202, 203, 201, 202, 203, 201, 101, 102, 201, 203, 102, 101, 202, 203, 201, 102, 101, 102, 202, 101, 203, 201, 202, 102, 101, 102, 101, 201, 202, 203, 201, 202, 203, 201, 101, 202, 203, 201], dtype=int32)
- vertex_index(vertex)int32...
- long_name :
- vertices index
array([2144930, 2144933, 2144934, 2144936, 2144938, 2144939, 2144940, 2144954, 2144955, 2144956, 2144957, 2144958, 2144959, 2144960, 2144961, 2144962, 2144963, 2144964, 2144969, 2144979, 2144984, 2144985, 2145003, 2145004, 2145005, 2145006, 2145007, 2145008, 2145009, 2145011, 2145012, 2145015, 2145021, 2145022, 2145023, 2145024, 2145025, 2145026, 2145027, 2145218, 2145219, 2145221, 2145223, 2145224, 2145225, 2145232, 2145238, 2145239, 2145240, 2145287], dtype=int32)
- edge_orientation(ne, vertex)int32...
- long_name :
- edge orientation
array([[ 1, 1, 1, ..., 1, 1, 1], [-1, -1, -1, ..., -1, -1, -1], [ 1, 1, 1, ..., 1, 1, 1], [-1, -1, -1, ..., -1, -1, -1], [-1, -1, -1, ..., -1, -1, -1], [ 1, 1, 1, ..., 1, 1, 1]], dtype=int32)
- edge_system_orientation(edge)int32...
- long_name :
- edge system orientation
array([-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1], dtype=int32)
- refin_c_ctrl(cell)int32...
- long_name :
- refinement control flag for cells
array([-4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4], dtype=int32)
- index_c_list(two_grf, cell_grf)int32...
- long_name :
- list of start and end indices for each refinement control level for cells
array([[-2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647], [-2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647]], dtype=int32)
- start_idx_c(max_chdom, cell_grf)int32...
- long_name :
- list of start indices for each refinement control level for cells
array([[20971521, 20971521, 20971521, 20971521, 20971521, 20971521, 20971521, 20971521, 20971521, 1, 1, 1, 1, 1]], dtype=int32)
- end_idx_c(max_chdom, cell_grf)int32...
- long_name :
- list of end indices for each refinement control level for cells
array([[20971520, 20971520, 20971520, 20971520, 20971520, 20971520, 20971520, 20971520, 20971520, 0, 0, 0, 0, 0]], dtype=int32)
- refin_e_ctrl(edge)int32...
- long_name :
- refinement control flag for edges
array([-8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8, -8], dtype=int32)
- index_e_list(two_grf, edge_grf)int32...
- long_name :
- list of start and end indices for each refinement control level for edges
array([[-2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647], [-2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647]], dtype=int32)
- start_idx_e(max_chdom, edge_grf)int32...
- long_name :
- list of start indices for each refinement control level for edges
array([[31457281, 31457281, 31457281, 31457281, 31457281, 31457281, 31457281, 31457281, 31457281, 31457281, 31457281, 31457281, 31457281, 31457281, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]], dtype=int32)
- end_idx_e(max_chdom, edge_grf)int32...
- long_name :
- list of end indices for each refinement control level for edges
array([[31457280, 31457280, 31457280, 31457280, 31457280, 31457280, 31457280, 31457280, 31457280, 31457280, 31457280, 31457280, 31457280, 31457280, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]], dtype=int32)
- refin_v_ctrl(vertex)int32...
- long_name :
- refinement control flag for vertices
array([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0], dtype=int32)
- index_v_list(two_grf, vert_grf)int32...
- long_name :
- list of start and end indices for each refinement control level for vertices
array([[-2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647], [-2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647, -2147483647]], dtype=int32)
- start_idx_v(max_chdom, vert_grf)int32...
- long_name :
- list of start indices for each refinement control level for vertices
array([[10485763, 10485763, 10485763, 10485763, 10485763, 10485763, 10485763, 10485763, 1, 1, 1, 1, 1]], dtype=int32)
- end_idx_v(max_chdom, vert_grf)int32...
- long_name :
- list of end indices for each refinement control level for vertices
array([[10485762, 10485762, 10485762, 10485762, 10485762, 10485762, 10485762, 10485762, 0, 0, 0, 0, 0]], dtype=int32)
- parent_edge_index(edge)int32...
- long_name :
- parent edge index
array([1607762, 1607765, 1607767, 1607762, 1607766, 1607766, 1607766, 1607767, 1607767, 1607779, 1607777, 1607778, 1607777, 1607778, 1607778, 1607779, 1607777, 1607779, 1607780, 1607777, 1607765, 1607780, 1607782, 1607778, 1607781, 1607781, 1607781, 1607782, 1607782, 1607766, 1607779, 1607783, 1607783, 1607783, 1607784, 1607787, 1607784, 1607767, 1607787, 1607797, 1607797, 1607814, 1607812, 1607813, 1607812, 1607813, 1607813, 1607814, 1607812, 1607814, 1607815, 1607812, 1607797, 1607815, 1607815, 1607781, 1607813, 1607816, 1607816, 1607816, 1607799, 1607818, 1607817, 1607819, 1607821, 1607820, 1607824, 1607819, 1607825, 1607825, 1607824, 1607824, 1607828, 1607826, 1607827, 1607826, 1607827, 1607827, 1607828, 1607826, 1607828, 1607783, 1607826, 1607824, 1607829, 1607827, 1607782, 1607829, 1607829, 1607830, 1607840, 1607977, 1607978, 1607981, 1607977, 1607980, 1607980, 1607981, 1607981, 1607957, 1607982, 1607994, 1607992, 1607993, 1607992, 1607993, 1607993, 1607994, 1607990, 1607816, 1607993, 1607981, 1607986, 1607994, 1607815, 1608035, 1607980, 1608035, 1607829], dtype=int32)
- child_edge_index(no, edge)int32...
- long_name :
- child edge index
array([[0, 0, 0, ..., 0, 0, 0], [0, 0, 0, ..., 0, 0, 0], [0, 0, 0, ..., 0, 0, 0], [0, 0, 0, ..., 0, 0, 0]], dtype=int32)
- child_edge_id(edge)int32...
- long_name :
- domain ID of child edge
array([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0], dtype=int32)
- parent_vertex_index(vertex)int32...
- long_name :
- parent vertex index
array([-1607762, 537170, 537171, -1607765, -1607766, -1607767, 537173, -1607777, -1607778, -1607779, 537178, 537179, -1607780, -1607781, -1607782, 537180, -1607783, -1607784, -1607787, 537186, -1607797, 537188, -1607812, -1607813, -1607814, 537192, -1607815, -1607816, -1607817, -1607819, 537193, -1607820, -1607825, -1607824, -1607826, -1607827, -1607828, 537198, -1607829, -1607977, -1607978, 537243, -1607980, -1607981, -1607982, -1607986, -1607992, -1607993, -1607994, -1608035], dtype=int32)
- cartesian_x_vertices(vertex)float64...
- long_name :
- vertex cartesian coordinate x on unit sp
- units :
- meters
array([0.575683, 0.574898, 0.576467, 0.574004, 0.575791, 0.576576, 0.576684, 0.574112, 0.574219, 0.575006, 0.573326, 0.575112, 0.573218, 0.573433, 0.574326, 0.573538, 0.575898, 0.57736 , 0.577468, 0.571536, 0.570749, 0.569961, 0.571644, 0.572539, 0.572431, 0.571751, 0.570856, 0.572645, 0.577787, 0.577682, 0.576896, 0.577001, 0.577575, 0.57679 , 0.576005, 0.575218, 0.57611 , 0.575323, 0.574431, 0.572854, 0.572065, 0.571961, 0.573643, 0.57275 , 0.571172, 0.570068, 0.571068, 0.571857, 0.570963, 0.574535])
- cartesian_y_vertices(vertex)float64...
- long_name :
- vertex cartesian coordinate y on unit sp
- units :
- meters
array([0.105629, 0.105036, 0.106223, 0.105639, 0.104433, 0.105026, 0.10383 , 0.104442, 0.103245, 0.103839, 0.103848, 0.102642, 0.105045, 0.10265 , 0.102047, 0.101452, 0.103236, 0.10562 , 0.104423, 0.105054, 0.104459, 0.103864, 0.103856, 0.103253, 0.104451, 0.102658, 0.103261, 0.102055, 0.100834, 0.102031, 0.101436, 0.100239, 0.103227, 0.102633, 0.102039, 0.101444, 0.100842, 0.100247, 0.10085 , 0.099659, 0.099063, 0.100261, 0.100254, 0.100857, 0.099666, 0.102666, 0.100864, 0.10146 , 0.102063, 0.099652])
- cartesian_z_vertices(vertex)float64...
- long_name :
- vertex cartesian coordinate z on unit sp
- units :
- meters
array([0.810822, 0.811456, 0.810187, 0.81201 , 0.8109 , 0.810265, 0.810343, 0.812088, 0.812165, 0.811533, 0.81272 , 0.81161 , 0.812642, 0.812796, 0.812242, 0.812872, 0.810977, 0.80963 , 0.809707, 0.813824, 0.814453, 0.815081, 0.813902, 0.81335 , 0.813273, 0.813979, 0.814531, 0.813426, 0.809935, 0.80986 , 0.810495, 0.810569, 0.809784, 0.810419, 0.811053, 0.811685, 0.811128, 0.81176 , 0.812317, 0.813576, 0.814204, 0.81413 , 0.812947, 0.813502, 0.814757, 0.815158, 0.814683, 0.814055, 0.814607, 0.812391])
- edge_middle_cartesian_x(edge)float64...
- long_name :
- prime edge center cartesian coordinate x on unit sphere
- units :
- meters
array([0.57529 , 0.574451, 0.575737, 0.576183, 0.576129, 0.575344, 0.576237, 0.57663 , 0.576521, 0.574166, 0.574613, 0.574559, 0.573719, 0.573773, 0.574666, 0.575059, 0.574505, 0.574952, 0.574058, 0.573611, 0.573665, 0.573272, 0.573826, 0.573879, 0.574273, 0.573379, 0.573486, 0.573932, 0.574719, 0.575452, 0.575845, 0.575398, 0.575505, 0.576291, 0.576913, 0.576968, 0.577022, 0.577414, 0.577076, 0.570355, 0.571143, 0.572092, 0.572485, 0.572038, 0.571698, 0.572145, 0.572932, 0.572879, 0.57159 , 0.571984, 0.571197, 0.570803, 0.57125 , 0.570409, 0.571304, 0.572592, 0.573039, 0.572986, 0.572198, 0.573092, 0.572825, 0.577734, 0.577342, 0.577289, 0.577394, 0.576948, 0.577629, 0.577183, 0.577236, 0.57713 , 0.576737, 0.576843, 0.575611, 0.575664, 0.576057, 0.575559, 0.575165, 0.57527 , 0.575716, 0.57645 , 0.576503, 0.576398, 0.576344, 0.575952, 0.574772, 0.574378, 0.574825, 0.573985, 0.574877, 0.576555, 0.577522, 0.572408, 0.572013, 0.573249, 0.573197, 0.572802, 0.573591, 0.573144, 0.572356, 0.571619, 0.571567, 0.571462, 0.57141 , 0.571015, 0.571515, 0.571909, 0.571804, 0.571357, 0.57112 , 0.572304, 0.572698, 0.572251, 0.57091 , 0.570462, 0.570515, 0.574929, 0.574483, 0.574037, 0.574089])
- edge_middle_cartesian_y(edge)float64...
- long_name :
- prime edge center cartesian coordinate y on unit sphere
- units :
- meters
array([0.105333, 0.105337, 0.105031, 0.10473 , 0.105328, 0.104734, 0.104131, 0.104428, 0.105625, 0.103843, 0.103542, 0.10414 , 0.104145, 0.103546, 0.102943, 0.10324 , 0.104739, 0.104437, 0.10504 , 0.105342, 0.104743, 0.104446, 0.102947, 0.102349, 0.102646, 0.103249, 0.102051, 0.10175 , 0.102344, 0.103537, 0.103834, 0.104136, 0.102939, 0.103533, 0.105921, 0.105323, 0.104725, 0.105022, 0.104127, 0.104162, 0.104757, 0.103555, 0.103852, 0.104153, 0.103257, 0.102956, 0.10355 , 0.104149, 0.104455, 0.104752, 0.104158, 0.10386 , 0.103559, 0.103563, 0.10296 , 0.102654, 0.102353, 0.102952, 0.102357, 0.101754, 0.104748, 0.101432, 0.101135, 0.101734, 0.100537, 0.100838, 0.102629, 0.10293 , 0.102332, 0.103529, 0.103232, 0.102035, 0.101742, 0.101143, 0.10144 , 0.10234 , 0.102043, 0.100846, 0.100544, 0.101738, 0.101139, 0.102336, 0.102935, 0.102637, 0.101746, 0.101448, 0.101147, 0.101151, 0.100548, 0.100541, 0.103825, 0.09996 , 0.099662, 0.099957, 0.100556, 0.100258, 0.100853, 0.101155, 0.100559, 0.099364, 0.099964, 0.101162, 0.101761, 0.101464, 0.100563, 0.100861, 0.102059, 0.10236 , 0.100265, 0.101158, 0.101456, 0.101757, 0.102662, 0.102964, 0.102364, 0.099949, 0.100251, 0.100552, 0.099953])
- edge_middle_cartesian_z(edge)float64...
- long_name :
- prime edge center cartesian coordinate z on unit sphere
- units :
- meters
array([0.811139, 0.811733, 0.810861, 0.810583, 0.810544, 0.811178, 0.810621, 0.810304, 0.810226, 0.812127, 0.811849, 0.811811, 0.812404, 0.812443, 0.811888, 0.811572, 0.811772, 0.811495, 0.812049, 0.812326, 0.812365, 0.812681, 0.812481, 0.812519, 0.812204, 0.812758, 0.812835, 0.812557, 0.811926, 0.811255, 0.810939, 0.811217, 0.811293, 0.81066 , 0.809908, 0.809948, 0.809987, 0.809669, 0.810025, 0.814767, 0.814139, 0.813626, 0.813311, 0.813588, 0.813941, 0.813665, 0.813035, 0.812996, 0.813863, 0.813549, 0.814178, 0.814492, 0.814217, 0.814806, 0.814255, 0.813388, 0.813112, 0.813073, 0.813703, 0.813149, 0.812957, 0.809898, 0.810215, 0.810178, 0.810252, 0.810532, 0.809822, 0.810102, 0.81014 , 0.810064, 0.810381, 0.810457, 0.811369, 0.811407, 0.811091, 0.811332, 0.811648, 0.811723, 0.811444, 0.810774, 0.810811, 0.810736, 0.810698, 0.811015, 0.811964, 0.812279, 0.812001, 0.812595, 0.812039, 0.810849, 0.809746, 0.813853, 0.814167, 0.813262, 0.813225, 0.813539, 0.81291 , 0.813187, 0.813816, 0.814481, 0.814444, 0.814369, 0.814331, 0.814645, 0.814406, 0.814093, 0.814017, 0.814293, 0.81472 , 0.813779, 0.813464, 0.813741, 0.814569, 0.814844, 0.814883, 0.812076, 0.812354, 0.812632, 0.812669])
- edge_dual_middle_cartesian_x(edge)float64...
- long_name :
- dual edge center cartesian coordinate x on unit sphere
- units :
- meters
array([0.57529 , 0.574451, 0.575737, 0.576183, 0.576129, 0.575344, 0.576237, 0.57663 , 0.576521, 0.574166, 0.574612, 0.574559, 0.573719, 0.573773, 0.574666, 0.575059, 0.574505, 0.574952, 0.574058, 0.573611, 0.573665, 0.573272, 0.573826, 0.573879, 0.574273, 0.573379, 0.573486, 0.573932, 0.574719, 0.575452, 0.575845, 0.575398, 0.575505, 0.576291, 0.576913, 0.576968, 0.577022, 0.577414, 0.577076, 0.570355, 0.571143, 0.572092, 0.572485, 0.572038, 0.571698, 0.572145, 0.572932, 0.572879, 0.57159 , 0.571984, 0.571197, 0.570803, 0.57125 , 0.570409, 0.571304, 0.572592, 0.573039, 0.572986, 0.572198, 0.573092, 0.572825, 0.577734, 0.577341, 0.577289, 0.577394, 0.576949, 0.577629, 0.577183, 0.577236, 0.57713 , 0.576737, 0.576843, 0.575611, 0.575664, 0.576057, 0.575558, 0.575165, 0.575271, 0.575716, 0.57645 , 0.576503, 0.576397, 0.576344, 0.575952, 0.574772, 0.574378, 0.574824, 0.573985, 0.574877, 0.576555, 0.577522, 0.572408, 0.572013, 0.573249, 0.573197, 0.572802, 0.573591, 0.573144, 0.572356, 0.571619, 0.571567, 0.571462, 0.57141 , 0.571016, 0.571515, 0.571909, 0.571804, 0.571357, 0.57112 , 0.572304, 0.572698, 0.572251, 0.57091 , 0.570462, 0.570515, 0.574929, 0.574483, 0.574037, 0.574089])
- edge_dual_middle_cartesian_y(edge)float64...
- long_name :
- dual edge center cartesian coordinate y on unit sphere
- units :
- meters
array([0.105333, 0.105337, 0.105031, 0.10473 , 0.105328, 0.104734, 0.104131, 0.104428, 0.105625, 0.103843, 0.103542, 0.10414 , 0.104145, 0.103546, 0.102943, 0.10324 , 0.104739, 0.104437, 0.10504 , 0.105342, 0.104743, 0.104446, 0.102947, 0.102349, 0.102646, 0.103249, 0.102051, 0.10175 , 0.102345, 0.103537, 0.103834, 0.104136, 0.102939, 0.103533, 0.105921, 0.105323, 0.104725, 0.105022, 0.104127, 0.104162, 0.104757, 0.103554, 0.103852, 0.104154, 0.103257, 0.102956, 0.10355 , 0.104149, 0.104455, 0.104752, 0.104158, 0.10386 , 0.103559, 0.103563, 0.10296 , 0.102654, 0.102353, 0.102951, 0.102357, 0.101754, 0.104748, 0.101432, 0.101135, 0.101734, 0.100537, 0.100838, 0.102629, 0.10293 , 0.102332, 0.103528, 0.103232, 0.102035, 0.101742, 0.101143, 0.10144 , 0.10234 , 0.102043, 0.100846, 0.100545, 0.101738, 0.101139, 0.102336, 0.102935, 0.102638, 0.101746, 0.101448, 0.101147, 0.101151, 0.100548, 0.100541, 0.103825, 0.09996 , 0.099662, 0.099957, 0.100556, 0.100258, 0.100853, 0.101155, 0.100559, 0.099364, 0.099964, 0.101162, 0.101761, 0.101464, 0.100563, 0.100861, 0.102059, 0.102361, 0.100265, 0.101158, 0.101456, 0.101758, 0.102662, 0.102964, 0.102364, 0.09995 , 0.100251, 0.100552, 0.099953])
- edge_dual_middle_cartesian_z(edge)float64...
- long_name :
- dual edge center cartesian coordinate z on unit sphere
- units :
- meters
array([0.811139, 0.811733, 0.810861, 0.810583, 0.810544, 0.811178, 0.810621, 0.810304, 0.810226, 0.812127, 0.811849, 0.811811, 0.812404, 0.812443, 0.811888, 0.811572, 0.811772, 0.811494, 0.812049, 0.812326, 0.812365, 0.812681, 0.812481, 0.812519, 0.812204, 0.812758, 0.812834, 0.812557, 0.811926, 0.811255, 0.810938, 0.811217, 0.811294, 0.81066 , 0.809908, 0.809948, 0.809987, 0.809669, 0.810025, 0.814767, 0.814139, 0.813626, 0.813311, 0.813588, 0.813941, 0.813665, 0.813035, 0.812996, 0.813863, 0.813549, 0.814178, 0.814492, 0.814217, 0.814806, 0.814255, 0.813388, 0.813112, 0.813073, 0.813703, 0.813149, 0.812957, 0.809898, 0.810215, 0.810178, 0.810252, 0.810532, 0.809822, 0.810102, 0.81014 , 0.810064, 0.810381, 0.810457, 0.811369, 0.811407, 0.811091, 0.811332, 0.811648, 0.811723, 0.811444, 0.810774, 0.810811, 0.810736, 0.810698, 0.811015, 0.811964, 0.812279, 0.812001, 0.812595, 0.812039, 0.810849, 0.809746, 0.813853, 0.814167, 0.813262, 0.813225, 0.813539, 0.81291 , 0.813187, 0.813816, 0.814481, 0.814444, 0.814369, 0.814331, 0.814645, 0.814407, 0.814093, 0.814017, 0.814293, 0.81472 , 0.813779, 0.813464, 0.813741, 0.814569, 0.814844, 0.814883, 0.812076, 0.812354, 0.812632, 0.812669])
- edge_primal_normal_cartesian_x(edge)float64...
- long_name :
- unit normal to the prime edge 3D vector, coordinate x
- units :
- meters
array([ 0.468375, -0.355597, -0.812663, 0.467708, 0.354673, -0.355383, -0.355169, 0.812079, 0.812056, -0.813874, 0.467961, 0.356092, -0.468627, -0.356801, -0.356589, 0.813292, -0.468501, 0.813269, 0.813851, -0.469167, -0.356305, -0.814455, -0.468088, -0.357298, 0.813897, -0.814478, -0.814501, 0.467548, 0.467421, -0.355879, 0.812686, -0.467834, 0.467294, 0.467167, -0.353962, -0.467581, -0.354458, 0.811471, 0.46704 , 0.470208, 0.470083, -0.35772 , 0.815058, -0.469418, -0.815661, 0.46888 , 0.468754, 0.357013, -0.815637, 0.357225, 0.357931, -0.816239, 0.469545, -0.358637, -0.358427, -0.815081, 0.468215, 0.357509, -0.358216, -0.358005, 0.469292, -0.811539, -0.355951, -0.465958, -0.465417, -0.812147, 0.811516, -0.466499, -0.355453, 0.354955, -0.812101, -0.812124, -0.466754, -0.356874, 0.812731, 0.356376, -0.813315, -0.813337, 0.466214, 0.356164, 0.466086, 0.466626, 0.355666, -0.812709, 0.357086, -0.81392 , 0.466881, -0.357794, -0.357583, 0.356662, -0.811493, 0.359209, -0.815729, 0.467137, 0.358502, -0.815127, 0.814524, -0.467676, -0.467804, 0.359916, -0.467931, 0.468469, 0.358923, -0.816285, -0.359419, 0.815706, 0.815684, -0.469007, 0.816308, -0.358713, 0.815104, -0.468342, 0.816262, -0.469671, -0.359132, -0.466342, 0.813942, -0.467009, -0.358292])
- edge_primal_normal_cartesian_y(edge)float64...
- long_name :
- unit normal to the prime edge 3D vector, coordinate y
- units :
- meters
array([-0.855416, -0.861102, -0.035696, -0.855571, 0.861221, -0.861246, -0.86139 , 0.035498, 0.035752, -0.035583, -0.85538 , 0.86127 , 0.855225, -0.861293, -0.861436, 0.035384, 0.855321, 0.03564 , 0.035839, 0.855165, -0.861126, -0.035782, 0.855284, -0.861458, 0.035326, -0.035525, -0.035267, -0.855341, -0.855438, -0.861413, 0.035441, 0.855476, -0.855534, -0.85563 , -0.861196, 0.855667, -0.861366, 0.035554, -0.855726, -0.854725, -0.85482 , -0.861173, 0.035725, 0.854975, -0.035667, -0.855034, -0.85513 , 0.86115 , -0.035926, 0.861007, 0.861031, -0.035868, -0.85488 , -0.861054, -0.861196, -0.035466, -0.855188, 0.861316, -0.861338, -0.86148 , -0.85507 , -0.034791, -0.861867, 0.855839, 0.855894, -0.034732, 0.035046, 0.855783, -0.861702, 0.861535, -0.035243, -0.034988, 0.855591, -0.861745, 0.034929, 0.86158 , -0.035127, -0.03487 , -0.855646, 0.861723, -0.855743, -0.855687, 0.861557, -0.035185, 0.861601, -0.035068, -0.855494, -0.861623, -0.861765, 0.861888, -0.0353 , 0.861664, -0.034886, -0.855301, 0.861643, -0.034947, 0.035008, 0.855245, 0.855149, 0.861683, 0.855052, -0.854996, 0.86136 , -0.035347, -0.861522, 0.035147, 0.035407, 0.854939, 0.035086, -0.861501, 0.035207, 0.855092, 0.035608, 0.854784, -0.861218, 0.855549, 0.034809, 0.855397, -0.861785])
- edge_primal_normal_cartesian_z(edge)float64...
- long_name :
- unit normal to the prime edge 3D vector, coordinate z
- units :
- meters
array([-0.221107, 0.363394, 0.58164 , -0.221917, -0.364012, 0.363261, 0.363128, -0.582467, -0.582484, 0.579951, -0.222121, -0.362509, 0.22131 , 0.361756, 0.361624, -0.580779, 0.221208, -0.580796, -0.579967, 0.220397, 0.362641, 0.579122, 0.222223, 0.360871, -0.579934, 0.579106, 0.579089, -0.223135, -0.223033, 0.362377, -0.581623, 0.222019, -0.222931, -0.222829, 0.364763, 0.221814, 0.363879, -0.583311, -0.222726, -0.219886, -0.219786, 0.361133, -0.578276, 0.220599, 0.57743 , -0.221512, -0.221411, -0.361888, 0.577447, -0.362018, -0.361263, 0.5766 , -0.2207 , 0.360507, 0.360377, 0.57826 , -0.222324, -0.361002, 0.360247, 0.360117, -0.220498, 0.583262, 0.361225, 0.224549, 0.22546 , 0.582419, -0.583278, 0.223638, 0.36211 , -0.362994, 0.582451, 0.582435, 0.223843, 0.360607, -0.581591, -0.361492, 0.580763, 0.580747, -0.224755, -0.361359, -0.224652, -0.22374 , -0.362244, 0.581607, -0.360739, 0.579918, -0.223946, 0.359986, 0.359854, -0.360474, 0.583294, -0.358475, 0.577381, -0.22415 , -0.359231, 0.578227, -0.579073, 0.223237, 0.223339, -0.357719, 0.223441, -0.222527, -0.359491, 0.576567, 0.358605, -0.577397, -0.577414, 0.221613, -0.576551, 0.359361, -0.578243, 0.222426, -0.576584, 0.2208 , 0.359621, 0.224858, -0.579902, 0.224048, 0.3591 ])
- edge_dual_normal_cartesian_x(edge)float64...
- long_name :
- unit normal to the dual edge 3D vector, coordinate x
- units :
- meters
array([-0.670572, -0.737263, -0.090035, -0.67027 , 0.736398, -0.736669, -0.736074, 0.08959 , 0.090492, -0.089122, -0.671441, 0.73694 , 0.67174 , -0.73721 , -0.736616, 0.088676, 0.671157, 0.089578, 0.090023, 0.671456, -0.737533, -0.089567, 0.672025, -0.736886, 0.088219, -0.088665, -0.087763, -0.67231 , -0.671726, -0.736346, 0.089133, 0.670856, -0.671141, -0.670555, -0.736126, 0.669683, -0.735802, 0.090047, -0.669968, -0.673498, -0.672918, -0.73807 , 0.089111, 0.672621, -0.088654, -0.672905, -0.672323, 0.737802, -0.089556, 0.738393, 0.738661, -0.0891 , -0.673202, -0.738927, -0.738338, -0.088209, -0.672608, 0.737479, -0.737747, -0.737155, -0.672039, -0.087339, -0.73483 , 0.670538, 0.670823, -0.086881, 0.088242, 0.670253, -0.735154, 0.735478, -0.088688, -0.087785, 0.671426, -0.735698, 0.087328, 0.736022, -0.087774, -0.08687 , -0.671711, 0.735427, -0.671125, -0.67084 , 0.73575 , -0.088231, 0.736293, -0.087317, -0.672011, -0.736563, -0.735969, 0.735103, -0.089145, 0.737101, -0.085946, -0.673178, 0.736832, -0.086403, 0.08686 , 0.672893, 0.673475, 0.737369, 0.674056, -0.673771, 0.738014, -0.087296, -0.737692, 0.086849, 0.087752, 0.673486, 0.086393, -0.737424, 0.087306, 0.67319 , 0.088198, 0.673782, -0.738604, 0.672296, 0.086413, 0.672595, -0.73624 ])
- edge_dual_normal_cartesian_y(edge)float64...
- long_name :
- unit normal to the dual edge 3D vector, coordinate y
- units :
- meters
array([-0.507117, 0.497401, 0.993828, -0.506981, -0.497196, 0.497279, 0.497156, -0.993899, -0.993763, 0.993957, -0.507547, -0.497363, 0.507684, 0.497446, 0.497323, -0.994027, 0.507401, -0.993893, -0.993822, 0.507538, 0.497485, 0.993887, 0.50783 , 0.497407, -0.994091, 0.994021, 0.994154, -0.507974, -0.507693, 0.497239, -0.993963, 0.507264, -0.507411, -0.507128, 0.497113, 0.506696, 0.497072, -0.993834, -0.506844, -0.508523, -0.508242, 0.497652, -0.993951, 0.508104, 0.994015, -0.508248, -0.507967, -0.497568, 0.993881, -0.497688, -0.497772, 0.993945, -0.508386, 0.497856, 0.497736, 0.994085, -0.508111, -0.49753 , 0.497614, 0.497492, -0.507821, 0.994234, 0.496947, 0.507138, 0.507284, 0.994296, -0.994102, 0.506991, 0.496989, -0.497031, 0.994033, 0.994165, 0.507556, 0.497158, -0.994228, -0.497199, 0.99416 , 0.994291, -0.507702, -0.497074, -0.50742 , -0.507274, -0.497115, 0.994096, -0.497283, 0.994223, -0.507838, 0.497368, 0.497243, -0.497032, 0.993969, -0.497538, 0.99441 , -0.508399, -0.497453, 0.994348, -0.994285, 0.508255, 0.508536, -0.497623, 0.508815, -0.508673, -0.497699, 0.994211, 0.497661, -0.99428 , -0.994148, 0.508529, -0.994342, 0.497576, -0.994217, 0.508392, -0.994079, 0.508667, 0.49782 , 0.507982, -0.994353, 0.508119, 0.497328])
- edge_dual_normal_cartesian_z(edge)float64...
- long_name :
- unit normal to the dual edge 3D vector, coordinate z
- units :
- meters
array([ 0.541448, 0.457203, -0.064803, 0.541949, -0.458818, 0.458292, 0.459381, 0.064335, 0.065161, -0.064085, 0.539966, -0.457766, -0.539464, 0.457241, 0.45833 , 0.063617, -0.540456, 0.064445, 0.064914, -0.539955, 0.456677, -0.064554, -0.538973, 0.457804, 0.063257, -0.063725, -0.062896, 0.538481, 0.539474, 0.458855, 0.063976, -0.540958, 0.540467, 0.541459, 0.459344, -0.542939, 0.459907, 0.064693, 0.54245 , 0.536474, 0.537468, 0.455626, 0.064193, -0.53797 , -0.063832, 0.537477, 0.538471, -0.456152, -0.064663, -0.455062, -0.454537, -0.064301, 0.536976, 0.454012, 0.455101, -0.063364, 0.537979, -0.456715, 0.45619 , 0.457279, 0.538963, -0.062216, 0.461593, -0.54147 , -0.54098 , -0.061857, 0.063041, -0.54196 , 0.461031, -0.460469, -0.063508, -0.062682, -0.539976, 0.45998 , 0.062323, -0.459418, -0.062789, -0.061962, 0.539484, -0.460505, 0.540478, 0.540969, -0.459943, -0.063149, -0.458892, -0.062429, 0.538983, 0.458367, 0.459454, -0.461067, -0.063867, -0.457316, -0.061342, 0.536994, -0.457842, -0.061705, 0.062067, -0.537487, -0.536491, -0.456791, -0.535496, 0.53599 , -0.455665, -0.062639, 0.456228, 0.062172, 0.063002, -0.536483, 0.061809, 0.456754, 0.062534, -0.536985, 0.06347 , -0.535981, 0.454576, -0.53849 , 0.061601, -0.537988, 0.458929])
- cell_circumcenter_cartesian_x(cell)float64...
- long_name :
- cartesian position of the prime cell circumcenter on the unit sphere, coordinate x
- units :
- meters
array([0.576012, 0.575462, 0.576355, 0.574441, 0.573891, 0.574784, 0.574677, 0.573783, 0.574333, 0.573547, 0.573998, 0.573654, 0.573761, 0.574548, 0.57557 , 0.575334, 0.57612 , 0.575227, 0.577139, 0.576796, 0.576905, 0.57221 , 0.571973, 0.57276 , 0.571866, 0.571078, 0.571315, 0.570528, 0.571423, 0.572867, 0.572317, 0.57321 , 0.573104, 0.572997, 0.577459, 0.577224, 0.577354, 0.577012, 0.577118, 0.575782, 0.57544 , 0.575546, 0.576332, 0.576227, 0.576568, 0.576462, 0.575676, 0.574654, 0.57489 , 0.574103, 0.574995, 0.576673, 0.577247, 0.573078, 0.573316, 0.572527, 0.571738, 0.571291, 0.571634, 0.571529, 0.571395, 0.572423, 0.572185, 0.572973, 0.572079, 0.570634, 0.571185, 0.574208, 0.574758, 0.573866])
- cell_circumcenter_cartesian_y(cell)float64...
- long_name :
- cartesian position of the prime cell circumcenter on the unit sphere, coordinate y
- units :
- meters
array([0.105043, 0.105019, 0.104416, 0.103855, 0.103831, 0.103228, 0.104425, 0.105028, 0.105053, 0.104458, 0.102634, 0.103261, 0.102063, 0.102658, 0.103822, 0.103252, 0.103846, 0.104449, 0.105009, 0.105637, 0.10444 , 0.10384 , 0.103269, 0.103864, 0.104467, 0.103872, 0.104443, 0.103848, 0.103245, 0.102666, 0.102642, 0.102039, 0.103237, 0.104434, 0.101421, 0.10085 , 0.102617, 0.103244, 0.102047, 0.101429, 0.102055, 0.100858, 0.101452, 0.102649, 0.102023, 0.10322 , 0.102626, 0.10146 , 0.102031, 0.101437, 0.100834, 0.100826, 0.103813, 0.10027 , 0.100841, 0.100246, 0.09965 , 0.101475, 0.100849, 0.102047, 0.100277, 0.101444, 0.100873, 0.101468, 0.102071, 0.10265 , 0.102674, 0.100239, 0.100262, 0.100865])
- cell_circumcenter_cartesian_z(cell)float64...
- long_name :
- cartesian position of the prime cell circumcenter on the unit sphere, coordinate z
- units :
- meters
array([0.810664, 0.811058, 0.810501, 0.811931, 0.812323, 0.811768, 0.811691, 0.812245, 0.811853, 0.812485, 0.8124 , 0.812562, 0.812639, 0.812008, 0.811135, 0.811375, 0.810742, 0.811298, 0.809866, 0.810029, 0.810107, 0.813507, 0.813746, 0.813116, 0.813668, 0.814297, 0.814058, 0.814686, 0.814136, 0.813193, 0.813583, 0.81303 , 0.812954, 0.812876, 0.810095, 0.810335, 0.81002 , 0.810184, 0.81026 , 0.811287, 0.811451, 0.811526, 0.810894, 0.810818, 0.810654, 0.810578, 0.811212, 0.812083, 0.811844, 0.812475, 0.811919, 0.810729, 0.809944, 0.813344, 0.813105, 0.813734, 0.814362, 0.814451, 0.814287, 0.814212, 0.814526, 0.813659, 0.813898, 0.813269, 0.813822, 0.814763, 0.814375, 0.81255 , 0.812158, 0.812714])
- title :
- ICON grid description
- institution :
- Max Planck Institute for Meteorology/Deutscher Wetterdienst
- source :
- git@git.mpimet.mpg.de:GridGenerator.git
- revision :
- d00fcac1f61fa16c686bfe51d1d8eddd09296cb5
- date :
- 20180529 at 222250
- user_name :
- Rene Redler (m300083)
- os_name :
- Linux 2.6.32-696.18.7.el6.x86_64 x86_64
- uuidOfHGrid :
- 0f1e7d66-637e-11e8-913b-51232bb4d8f9
- grid_mapping_name :
- lat_long_on_sphere
- crs_id :
- urn:ogc:def:cs:EPSG:6.0:6422
- crs_name :
- Spherical 2D Coordinate System
- ellipsoid_name :
- Sphere
- semi_major_axis :
- 6371229.0
- inverse_flattening :
- 0.0
- grid_level :
- 9
- grid_root :
- 2
- grid_ID :
- 1
- parent_grid_ID :
- 0
- no_of_subgrids :
- 1
- start_subgrid_id :
- 1
- max_childdom :
- 1
- boundary_depth_index :
- 0
- rotation_vector :
- [0. 0. 0.]
- grid_geometry :
- 1
- grid_cell_type :
- 3
- mean_edge_length :
- 7510.64679407352
- mean_dual_edge_length :
- 4336.344345177032
- mean_cell_area :
- 24323517.809282698
- mean_dual_cell_area :
- 48647026.33989711
- domain_length :
- 40031612.44147649
- domain_height :
- 40031612.44147649
- sphere_radius :
- 6371229.0
- domain_cartesian_center :
- [0. 0. 0.]
- centre :
- 252
- rotation :
- 37deg around z-axis
- coverage :
- global
- symmetry :
- along equator
- topography :
- modified SRTM30
- subcentre :
- 1
- number_of_grid_used :
- 15
- history :
- Thu Aug 16 11:05:44 2018: ncatted -O -a ICON_grid_file_uri,global,m,c,http://icon-downloads.mpimet.mpg.de/grids/public/mpim/0015/icon_grid_0015_R02B09_G.nc icon_grid_0015_R02B09_G.nc test.nc Wed May 30 08:50:27 2018: ncatted -a centre,global,c,i,252 -a rotation,global,c,c,37deg around z-axis -a coverage,global,c,c,global -a symmetry,global,c,c,along equator -a topography,global,c,c,modified SRTM30 -a subcentre,global,c,i,1 -a number_of_grid_used,global,c,i,15 -a ICON_grid_file_uri,global,c,c,http://icon-downloads.mpimet.mpg.de/grids/public/icon_grid_0015_R02B09_G.nc Earth_Global_IcosSymmetric_4932m_rotatedZ37d_modified_srtm30_1min.nc icon_grid_0015_R02B09_G.nc /mnt/lustre01/work/mh0287/users/rene/GridGenerator/build/x86_64-unknown-linux-gnu/bin/grid_command
- ICON_grid_file_uri :
- http://icon-downloads.mpimet.mpg.de/grids/public/mpim/0015/icon_grid_0015_R02B09_G.nc
- NCO :
- netCDF Operators version 4.7.5 (Homepage = http://nco.sf.net, Code = http://github.com/nco/nco)
%matplotlib inline
import matplotlib.pylab as plt
We create the artificially generated field:
vlon = np.rad2deg(new_grid.vlon)
vlat = np.rad2deg(new_grid.vlat)
voc = new_grid.vertex_of_cell.T.values - 1
test_field = np.rad2deg(new_grid.clon)
fig, ax = plt.subplots(figsize=(10, 6))
im = plt.tripcolor(vlon, vlat, voc, test_field, cmap=plt.cm.get_cmap("seismic"))
cbar = fig.colorbar(im)
cbar.set_label("arb. unit")
ax.set_title("Testfield")
ax.set_xlabel("Longitude / deg")
ax.set_ylabel("Latitude / deg")
plt.show()
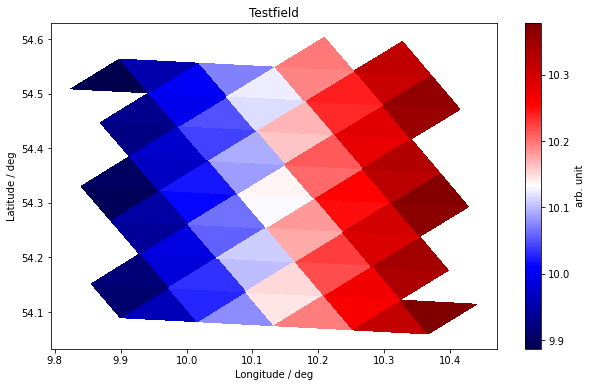
We just copied the function derivative()
from Part 2:
def derivative(grid, data):
neighbors = (grid.neighbor_cell_index.values - 1).T
valid = np.all(neighbors >= 0, axis=-1)
cell_lon = np.rad2deg(new_grid.clon.values[valid])
cell_lat = np.rad2deg(new_grid.clat.values[valid])
neighbors_lon = np.rad2deg(new_grid.clon.values[neighbors[valid]])
neighbors_lat = np.rad2deg(new_grid.clat.values[neighbors[valid]])
p = data.values[..., neighbors[valid]]
ones = np.ones_like(neighbors_lon)
A = np.stack(
(
ones,
neighbors_lon - cell_lon[:, np.newaxis],
neighbors_lat - cell_lat[:, np.newaxis],
),
axis=2,
)
A_inv = np.linalg.inv(A)
alpha, beta, gamma = np.einsum("...ij,...j->i...", A_inv, p)
return alpha, beta, gamma, valid
We plug in our test_field
and calculate the derivatives in both directions:
test_0, dtestdlon, dtestdlat, valid = derivative(new_grid, test_field)
Let’s plot it and we see that we do not see much … But wait, eureka ! Take a look at the range of the colorbar. We are at \(1\mathrm{e}{-13}\)…
voc_valid = voc[valid]
fig, ax = plt.subplots(figsize=(10, 6))
im = plt.tripcolor(vlon, vlat, voc_valid, dtestdlon, cmap=plt.cm.get_cmap("seismic"))
cbar = fig.colorbar(im)
cbar.set_label("arb. unit")
ax.set_title("Longitudinal Derivative of Testfield")
ax.set_xlabel("Longitude / deg")
ax.set_ylabel("Latitude / deg")
plt.show()
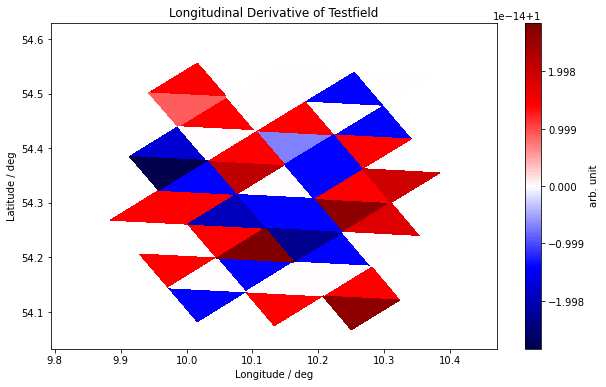
…so we simply round to the 12th digit after the decimal point and get a white area with the corresponding color scheme. What we get is a derivative approximately equal to 1
for the whole area of interest.
dtestdlon_rounded = np.round(dtestdlon, 12)
fig, ax = plt.subplots(figsize=(10, 6))
im = plt.tripcolor(
vlon, vlat, voc_valid, dtestdlon_rounded, cmap=plt.cm.get_cmap("seismic")
)
cbar = fig.colorbar(im)
cbar.set_label("arb. unit")
ax.set_title("Longitudinal Derivative of Testfield")
ax.set_xlabel("Longitude / deg")
ax.set_ylabel("Latitude / deg")
plt.show()
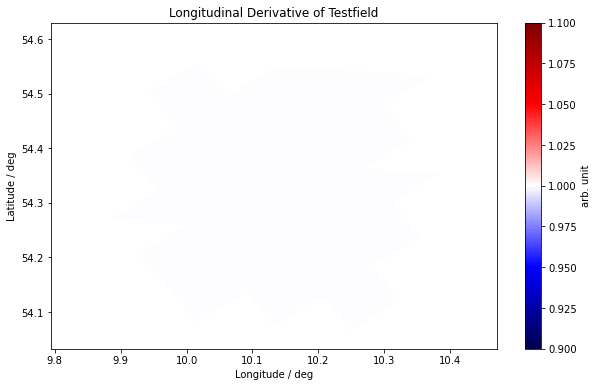
This is exactly what we expected. Because, if you have been paying attention, you will have seen that we have chosen as test_field
the longitudinal coordinate of the corresponding cell, namely test_field = np.rad2deg(new_grid.clon)
. This means that for every 1 deg
change in position along the west-east axis, the value of our test field has changed by exactly 1
. The corresponding derivation along the chosen axis then inevitably results in 1
- voilà !
The same can of course still be done and checked along the latitudinal axis. For this purpose, a corresponding test_field
must be generated, but we leave this to you as a small task. Enjoy !